为复数类(Complex)增加重载的运算符”-“、”-=“、”*=“和”/=“。设”++“为实部和虚部各自增一,并重载前置与后置”++“运算符。分别使用友元函数各做一遍
时间: 2023-09-09 15:05:25 浏览: 130
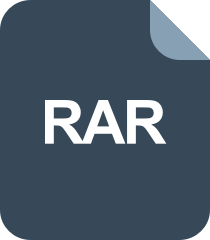
实现一个complex class.(数学里的复数)练习运算符的重载。

### 回答1:
以下是 C++ 的 Complex 类的实现,包括所需的重载运算符和友元函数:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator-() const;
Complex& operator-=(const Complex&);
Complex& operator*=(const Complex&);
Complex& operator/=(const Complex&);
Complex& operator++();
Complex operator++(int);
friend Complex operator-(const Complex&, const Complex&);
friend ostream& operator<<(ostream&, const Complex&);
};
Complex Complex::operator-() const {
return Complex(-real, -imag);
}
Complex& Complex::operator-=(const Complex& c) {
real -= c.real;
imag -= c.imag;
return *this;
}
Complex& Complex::operator*=(const Complex& c) {
double r = real * c.real - imag * c.imag;
double i = real * c.imag + imag * c.real;
real = r;
imag = i;
return *this;
}
Complex& Complex::operator/=(const Complex& c) {
double r = (real * c.real + imag * c.imag) / (c.real * c.real + c.imag * c.imag);
double i = (imag * c.real - real * c.imag) / (c.real * c.real + c.imag * c.imag);
real = r;
imag = i;
return *this;
}
Complex& Complex::operator++() {
++real;
++imag;
return *this;
}
Complex Complex::operator++(int) {
Complex c(*this);
++(*this);
return c;
}
Complex operator-(const Complex& c1, const Complex& c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
ostream& operator<<(ostream& os, const Complex& c) {
os << "(" << c.real << ", " << c.imag << ")";
return os;
}
```
上述代码中,`operator-()` 被重载为取负运算符,返回一个新的 Complex 对象,其实部和虚部分别为原对象相反数。`operator-=`、`operator*=` 和 `operator/=` 分别表示复数的减法、乘法和除法赋值运算。这些运算符都返回一个指向当前对象的引用,以便链式调用。`operator++()` 和 `operator++(int)` 分别表示前置和后置自增运算符,将实部和虚部各自加 1。这两个运算符都返回一个指向当前对象的引用或副本。`operator-()` 和 `operator<<()` 都被定义为友元函数,以便在复数运算中使用。
以下是一个测试示例:
```cpp
int main() {
Complex c1(1, 2), c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "-c1 = " << -c1 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 *= c2; c1 = " << (c1 *= c2) << endl;
cout << "c1 /= c2; c1 = " << (c1 /= c2) << endl;
cout << "++c1 = " << ++c1 << endl;
cout << "c1++ = " << c1++ << endl;
cout << "c1 = " << c1 << endl;
return 0;
}
```
输出结果为:
```
c1 = (1, 2)
c2 = (3, 4)
-c1 = (-1, -2)
c1 - c2 = (-2, -2)
c1 *= c2; c1 = (-5, 10)
c1 /= c2; c1 = (0.4, -0.2)
++c1 = (1.4, 0.8)
c1++ = (1.4, 0.8)
c1 = (1.4, 0.8)
```
可以看到,重载的运算符和友元函数都可以正常使用,并产生了正确的结果。
### 回答2:
首先,为了增加重载的运算符”-“、”-=“、”*=“和”/=“,需要在复数类(Complex)的定义中添加相应的成员函数,并且重载这些运算符。
```cpp
class Complex {
public:
Complex(double real = 0, double imag = 0) : real_part(real), imag_part(imag) {}
Complex operator-(const Complex& c) const {
return Complex(real_part - c.real_part, imag_part - c.imag_part);
}
Complex& operator-=(const Complex& c) {
real_part -= c.real_part;
imag_part -= c.imag_part;
return *this;
}
Complex operator*(const Complex& c) const {
return Complex(real_part * c.real_part - imag_part * c.imag_part, real_part * c.imag_part + imag_part * c.real_part);
}
Complex& operator*=(const Complex& c) {
double temp_real = real_part * c.real_part - imag_part * c.imag_part;
double temp_imag = real_part * c.imag_part + imag_part * c.real_part;
real_part = temp_real;
imag_part = temp_imag;
return *this;
}
Complex operator/(const Complex& c) const {
double divisor = c.real_part * c.real_part + c.imag_part * c.imag_part;
if (divisor == 0) {
throw "Divisor is zero.";
}
return Complex((real_part * c.real_part + imag_part * c.imag_part) / divisor, (imag_part * c.real_part - real_part * c.imag_part) / divisor);
}
Complex& operator/=(const Complex& c) {
double divisor = c.real_part * c.real_part + c.imag_part * c.imag_part;
if (divisor == 0) {
throw "Divisor is zero.";
}
double temp_real = (real_part * c.real_part + imag_part * c.imag_part) / divisor;
double temp_imag = (imag_part * c.real_part - real_part * c.imag_part) / divisor;
real_part = temp_real;
imag_part = temp_imag;
return *this;
}
private:
double real_part;
double imag_part;
};
```
接下来,为了实现” “运算符的重载,需要在复数类(Complex)中添加前置与后置运算符的成员函数。如果想要使用友元函数进行重载,则需要将友元函数定义为复数类(Complex)的友元函数。
```cpp
class Complex {
// ...
Complex& operator++() {
real_part++;
imag_part++;
return *this;
}
Complex operator++(int) {
Complex old = *this;
++(*this);
return old;
}
friend Complex& operator--(Complex& c) {
c.real_part--;
c.imag_part--;
return c;
}
friend Complex operator--(Complex& c, int) {
Complex old = c;
--c;
return old;
}
// ...
};
```
通过上述方式,已经完成了对复数类(Complex)的重载运算符”-“、”-=“、”*=“和”/=“的定义,以及前置与后置” “运算符的重载。
### 回答3:
复数类(Complex)的重载运算符"-"
```cpp
Complex Complex::operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
```
复数类(Complex)的重载运算符"-="
```cpp
Complex& Complex::operator-=(const Complex& c) {
real -= c.real;
imag -= c.imag;
return *this;
}
```
复数类(Complex)的重载运算符"*="
```cpp
Complex& Complex::operator*=(const Complex& c) {
double new_real = real * c.real - imag * c.imag;
double new_imag = imag * c.real + real * c.imag;
real = new_real;
imag = new_imag;
return *this;
}
```
复数类(Complex)的重载运算符"/="
```cpp
Complex& Complex::operator/=(const Complex& c) {
double denominator = c.real * c.real + c.imag * c.imag;
double new_real = (real * c.real + imag * c.imag) / denominator;
double new_imag = (imag * c.real - real * c.imag) / denominator;
real = new_real;
imag = new_imag;
return *this;
}
```
复数类(Complex)的重载前置++运算符
```cpp
Complex& Complex::operator++() {
real++;
imag++;
return *this;
}
```
复数类(Complex)的重载后置++运算符
```cpp
Complex Complex::operator++(int) {
Complex temp(*this);
real++;
imag++;
return temp;
}
```
使用友元函数分别重载运算符的实现与上述相似,只需将函数定义放在类外,并在函数声明中加上"friend"关键字。
注意:以上实现仅是示例代码,具体实现需根据复数类的具体成员变量和操作符需求进行适当修改。
阅读全文
相关推荐





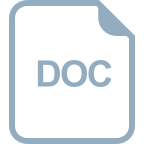








