用Java编写复数类的加减乘除运算
时间: 2023-05-29 16:04:10 浏览: 95
复数类(Complex)的代码如下:
```
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
return new Complex(this.real + other.real, this.imag + other.imag);
}
public Complex subtract(Complex other) {
return new Complex(this.real - other.real, this.imag - other.imag);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
public double getReal() {
return this.real;
}
public double getImag() {
return this.imag;
}
public String toString() {
return this.real + " + " + this.imag + "i";
}
}
```
使用示例:
```
Complex a = new Complex(3, 4);
Complex b = new Complex(1, 2);
Complex c = a.add(b);
System.out.println(c); // 4.0 + 6.0i
Complex d = a.subtract(b);
System.out.println(d); // 2.0 + 2.0i
Complex e = a.multiply(b);
System.out.println(e); // -5.0 + 10.0i
Complex f = a.divide(b);
System.out.println(f); // 2.2 - 0.4i
```
阅读全文
相关推荐
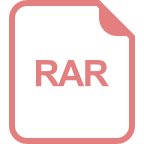
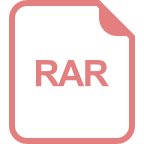
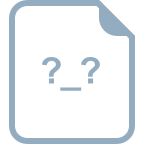















