如何在Java中实现复数的基本运算(加减乘除)并编写相关实验代码?
时间: 2024-10-13 11:19:04 浏览: 62
在Java中,我们可以创建一个名为ComplexNumber的类来表示复数,并提供基本的加、减、乘、除操作。以下是一个简单的实现:
```java
public class ComplexNumber {
private double real;
private double imaginary;
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// Getter and Setter methods
public double getReal() { return real; }
public void setReal(double real) { this.real = real; }
public double getImaginary() { return imaginary; }
public void setImaginary(double imaginary) { this.imaginary = imaginary; }
// Addition of two complex numbers
public ComplexNumber add(ComplexNumber other) {
double newReal = this.real + other.getReal();
double newImaginary = this.imaginary + other.getImaginary();
return new ComplexNumber(newReal, newImaginary);
}
// Subtraction
public ComplexNumber subtract(ComplexNumber other) {
double newReal = this.real - other.getReal();
double newImaginary = this.imaginary - other.getImaginary();
return new ComplexNumber(newReal, newImaginary);
}
// Multiplication
public ComplexNumber multiply(ComplexNumber other) {
double realPart = (this.real * other.getReal()) - (this.imaginary * other.getImaginary());
double imagPart = (this.real * other.getImaginary()) + (this.imaginary * other.getReal());
return new ComplexNumber(realPart, imagPart);
}
// Division
public ComplexNumber divide(ComplexNumber other) {
double denominator = other.getReal() * other.getReal() + other.getImaginary() * other.getImaginary();
double realPart = ((this.real * other.getReal()) + (this.imaginary * other.getImaginary())) / denominator;
double imagPart = ((this.imaginary * other.getReal()) - (this.real * other.getImaginary())) / denominator;
return new ComplexNumber(realPart, imagPart);
}
@Override
public String toString() {
return "Complex{" +
"real=" + real +
", imaginary=" + imaginary +
'}';
}
}
// 使用示例
public static void main(String[] args) {
ComplexNumber c1 = new ComplexNumber(2, 3);
ComplexNumber c2 = new ComplexNumber(4, -5);
System.out.println("c1: " + c1);
System.out.println("c2: " + c2);
ComplexNumber sum = c1.add(c2);
System.out.println("Sum: " + sum);
ComplexNumber difference = c1.subtract(c2);
System.out.println("Difference: " + difference);
ComplexNumber product = c1.multiply(c2);
System.out.println("Product: " + product);
ComplexNumber quotient = c1.divide(c2);
if (quotient.getDenominator() != 0) {
System.out.println("Quotient: " + quotient);
} else {
System.out.println("Division by zero is not allowed.");
}
}
```
在这个例子中,我们首先定义了一个ComplexNumber类,包含了复数的实部和虚部,以及对应的操作方法。然后在main函数中展示了如何创建复数对象,并进行基本运算。
阅读全文
相关推荐
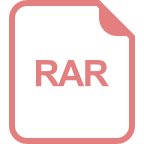
















