java编写一个复数类complex
时间: 2023-05-31 17:18:18 浏览: 1004
### 回答1:
复数类complex是一个用Java编写的类,用于表示复数。它包含两个实例变量,一个表示实部,一个表示虚部。它还包含一些方法,用于执行各种复数运算,如加法、减法、乘法和除法。
以下是一个简单的复数类complex的示例代码:
```
public class complex {
private double real;
private double imag;
public complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public complex add(complex other) {
return new complex(this.real + other.real, this.imag + other.imag);
}
public complex subtract(complex other) {
return new complex(this.real - other.real, this.imag - other.imag);
}
public complex multiply(complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new complex(real, imag);
}
public complex divide(complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new complex(real, imag);
}
public String toString() {
if (imag >= 0) {
return real + "+" + imag + "i";
} else {
return real + "-" + (-imag) + "i";
}
}
}
```
在这个示例中,我们定义了一个复数类complex,它有两个实例变量real和imag,分别表示实部和虚部。我们还定义了四个方法add、subtract、multiply和divide,用于执行加法、减法、乘法和除法运算。最后,我们还定义了一个toString方法,用于将复数转换为字符串表示形式。
使用这个复数类complex,我们可以轻松地执行各种复数运算,例如:
```
complex a = new complex(1, 2);
complex b = new complex(3, 4);
complex c = a.add(b);
System.out.println(c); // 输出:4+6i
complex d = a.multiply(b);
System.out.println(d); // 输出:-5+10i
```
这个示例演示了如何创建两个复数a和b,然后使用add和multiply方法执行加法和乘法运算。最后,我们使用toString方法将结果转换为字符串并输出。
### 回答2:
Java编写一个复数类complex
在数学中,复数是一种由实部和虚部组成的数。为了在Java中表示复数,我们可以创建一个复数类complex。首先,让我们定义一个复数类,它包括实部和虚部。我们可以使用双精度浮点数double来表示实部和虚部。
public class Complex{
private double real;
private double imaginary;
}
现在我们需要为复数类创建构造函数,以便它可以从实部和虚部创建一个复数对象。
public Complex(double real, double imaginary){
this.real = real;
this.imaginary = imaginary;
}
让我们为复数类创建一些实用的方法。要打印一个复数,我们可以使用toString方法。
public String toString(){
return "(" + real + " + " + imaginary + "i)";
}
我们还可以为复数类提供加、减、乘和除的运算。
public Complex add(Complex other){
return new Complex(real + other.real, imaginary + other.imaginary);
}
public Complex subtract(Complex other){
return new Complex(real - other.real, imaginary - other.imaginary);
}
public Complex multiply(Complex other){
double real = this.real * other.real - this.imaginary * other.imaginary;
double imaginary = this.real * other.imaginary + this.imaginary * other.real;
return new Complex(real, imaginary);
}
public Complex divide(Complex other){
double denominator = other.real * other.real + other.imaginary * other.imaginary;
double real = (this.real * other.real + this.imaginary * other.imaginary) / denominator;
double imaginary = (this.imaginary * other.real - this.real * other.imaginary) / denominator;
return new Complex(real, imaginary);
}
我们还可以为复数类提供其他实用的方法,例如求幅度和相位等。
public double magnitude(){
return Math.sqrt(real * real + imaginary * imaginary);
}
public double phase(){
return Math.atan2(imaginary, real);
}
这些方法将使我们能够轻松处理复数。以下是使用复数类的示例代码:
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex c3 = c1.add(c2);
System.out.println(c1 + " + " + c2 + " = " + c3);
复数类Complex使我们能够更轻松地处理复杂的数学计算。它是Java中强大的数学类库的一部分。
### 回答3:
Java编写一个复数类complex
复数可以表示为实部和虚部之间的一对数字,通常表示为a+bi的形式。在Java中,我们可以使用一个类来表示复数,让我们称之为complex类。
1. 创建一个Complex类
使用Java编写程序时,首先需要创建一个类。
public class Complex{
private double real;
private double imaginary;
}
在此代码中,我们声明了两个私有实例变量 real 和 imaginary,它们分别用于存储复数的实部和虚部。
2. 构造函数
为了实例化一个对象,我们需要一个构造函数。
public Complex(double real, double imaginary){
this.real = real;
this.imaginary = imaginary;
}
这个构造函数需要传递两个值,即实部和虚部。
3. 获取函数
为了获得实部和虚部,我们需要编写两个成员函数。
public double getReal(){
return real;
}
public double getImaginary(){
return imaginary;
}
这些函数只需返回实例变量的值即可。
4. 加法
在复数类中,我们需要实现加法操作。
public Complex add(Complex other){
double newReal = real + other.real;
double newImaginary = imaginary + other.imaginary;
return new Complex(newReal,newImaginary);
}
这个函数接受另一个复数作为参数,并返回一个新的Complex对象,包含两个复数之和。在这个函数中,我们简单地将两个复数实部和虚部分别加起来。
5. 减法
除了加法,我们还需要实现减法操作。
public Complex subtract(Complex other){
double newReal = real - other.real;
double newImaginary = imaginary - other.imaginary;
return new Complex(newReal,newImaginary);
}
这个函数与add函数非常相似,只需将每个复数的实部和虚部分别减去即可。
6. 乘法
复数的乘法涉及到计算公式(a+bi)*(c+di)。
public Complex multiply(Complex other){
double newReal = real*other.real - imaginary*other.imaginary;
double newImaginary = real*other.imaginary + imaginary*other.real;
return new Complex(newReal,newImaginary);
}
7. 打印
最后,我们还需要添加一个方法,用于打印复数。
public void print(){
System.out.println(real + " + " + imaginary + "i");
}
这个函数只需将实部和虚部作为字符串打印出来即可。
现在我们已经成功地创建了一个复数类,它包括构造函数、成员变量、加减乘函数和打印函数。我们可以使用这个类来创建任意数量的复数对象,并对它们进行各种计算。
相关推荐
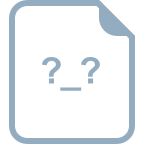
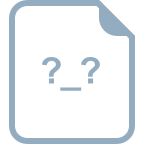















