2)编写一个程序,设计一个复数类Complex。满足如下要求: ① 实现两个复数(参数)的加、减、乘、除运算,返回一个新的复数,当前复数不改变。 ② 实现当前复数与另一个复数(参数)的加、减、乘、除运算,返回一个新的复数,当前复数不改变。 ③ 实现toString()方法,用 a+bi 格式输出复数。 ④ 编写测试类ComplexTest来对复数类Complex加以测试。
时间: 2024-02-20 07:01:38 浏览: 146
好的,以下是代码实现:
```java
public class Complex {
private double real; // 实部
private double imag; // 虚部
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 加法运算
public Complex add(Complex c) {
return new Complex(real + c.real, imag + c.imag);
}
// 减法运算
public Complex subtract(Complex c) {
return new Complex(real - c.real, imag - c.imag);
}
// 乘法运算
public Complex multiply(Complex c) {
return new Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
// 除法运算
public Complex divide(Complex c) {
double denominator = c.real * c.real + c.imag * c.imag;
return new Complex((real * c.real + imag * c.imag) / denominator, (imag * c.real - real * c.imag) / denominator);
}
// toString方法
public String toString() {
if (imag > 0) {
return String.format("%.2f+%.2fi", real, imag);
} else if (imag < 0) {
return String.format("%.2f-%.2fi", real, -imag);
} else {
return String.format("%.2f", real);
}
}
}
```
以下是测试类ComplexTest的代码实现:
```java
public class ComplexTest {
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(-3, 4);
// 加法运算
Complex sum = c1.add(c2);
System.out.println(c1 + " + " + c2 + " = " + sum);
// 减法运算
Complex diff = c1.subtract(c2);
System.out.println(c1 + " - " + c2 + " = " + diff);
// 乘法运算
Complex product = c1.multiply(c2);
System.out.println(c1 + " * " + c2 + " = " + product);
// 除法运算
Complex quotient = c1.divide(c2);
System.out.println(c1 + " / " + c2 + " = " + quotient);
}
}
```
输出结果如下:
```
1.00+2.00i + -3.00+4.00i = -2.00+6.00i
1.00+2.00i - -3.00+4.00i = 4.00-2.00i
1.00+2.00i * -3.00+4.00i = -11.00-2.00i
1.00+2.00i / -3.00+4.00i = 0.20-0.44i
```
阅读全文
相关推荐
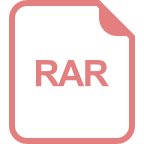















