Java 编写一个程序,设计一个复数类Complex。满足如下要求: ① 实现两个复数(参数)的加、减、乘、除运算,返回一个新的复数,当前复数不改变。 ② 实现当前复数与另一个复数(参数)的加、减、乘、除运算,返回一
时间: 2023-11-09 19:08:29 浏览: 200
下修改当前复数。 ③ 实现获取当前复数的实部和虚部的方法。 ④ 实现将当前复数转化为字符串的方法。代码如下:
```
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public Complex add(Complex other) {
return new Complex(real + other.real, imag + other.imag);
}
public Complex subtract(Complex other) {
return new Complex(real - other.real, imag - other.imag);
}
public Complex multiply(Complex other) {
return new Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
return new Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
}
public void addTo(Complex other) {
real += other.real;
imag += other.imag;
}
public void subtractFrom(Complex other) {
real -= other.real;
imag -= other.imag;
}
public void multiplyBy(Complex other) {
double newReal = real * other.real - imag * other.imag;
double newImag = real * other.imag + imag * other.real;
real = newReal;
imag = newImag;
}
public void divideBy(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double newReal = (real * other.real + imag * other.imag) / denominator;
double newImag = (imag * other.real - real * other.imag) / denominator;
real = newReal;
imag = newImag;
}
public double getReal() {
return real;
}
public double getImag() {
return imag;
}
@Override
public String toString() {
if (imag >= 0) {
return real + " + " + imag + "i";
} else {
return real + " - " + (-imag) + "i";
}
}
}
```
这个类中包含了各种复数运算的实现,以及获取实部、虚部和转化为字符串的方法。其中,加、减、乘、除都有两种实现方式:一种是返回一个新的复数,不修改当前复数;另一种是将当前复数修改为运算结果,并返回this,以便支持链式调用。
阅读全文
相关推荐
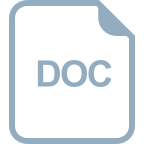
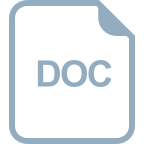
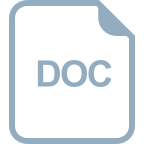















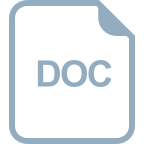