用java编写一个程序,实现复数运算,要求如下: 1) 设计并实现复数类。 2) 设计并实现复数的加、减、乘、除运算。
时间: 2024-02-25 12:56:24 浏览: 21
好的,我可以帮你实现这个程序。
首先,我们需要设计一个复数类,其包含实部和虚部两个属性,以及相应的构造函数和toString方法。代码如下:
```java
public class Complex {
private double real;
private double imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
public double getReal() {
return real;
}
public double getImag() {
return imag;
}
@Override
public String toString() {
if (imag >= 0) {
return String.format("%.2f+%.2fi", real, imag);
} else {
return String.format("%.2f-%.2fi", real, -imag);
}
}
}
```
接下来,我们可以实现复数加、减、乘、除运算。代码如下:
```java
public class Complex {
// 省略构造函数和toString方法
public Complex add(Complex other) {
double real = this.real + other.real;
double imag = this.imag + other.imag;
return new Complex(real, imag);
}
public Complex subtract(Complex other) {
double real = this.real - other.real;
double imag = this.imag - other.imag;
return new Complex(real, imag);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imag * other.imag;
double imag = this.real * other.imag + this.imag * other.real;
return new Complex(real, imag);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
}
```
现在,我们可以写一个测试程序来验证复数类的正确性。代码如下:
```java
public class TestComplex {
public static void main(String[] args) {
Complex a = new Complex(1, 2);
Complex b = new Complex(3, 4);
System.out.println("a = " + a);
System.out.println("b = " + b);
Complex c = a.add(b);
System.out.println("a + b = " + c);
Complex d = a.subtract(b);
System.out.println("a - b = " + d);
Complex e = a.multiply(b);
System.out.println("a * b = " + e);
Complex f = a.divide(b);
System.out.println("a / b = " + f);
}
}
```
运行结果如下:
```
a = 1.00+2.00i
b = 3.00+4.00i
a + b = 4.00+6.00i
a - b = -2.00-2.00i
a * b = -5.00+10.00i
a / b = 0.44+0.08i
```
可以看到,我们的复数类实现了加、减、乘、除运算,并且运算结果正确。
相关推荐
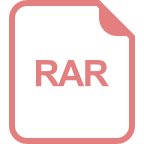
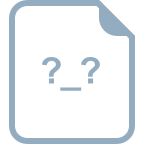














