java复数类的实现及运算实验总结
时间: 2024-01-22 19:03:36 浏览: 64
Java复数类的实现及运算实验总结:
1. 复数类的实现
为了表示复数,我们可以创建一个名为Complex的类。该类应该有两个实例变量:一个表示实部,另一个表示虚部。我们还需要实现一些方法来执行基本的数学运算,如加法、减法、乘法和除法。
下面是一个简单的Java复数类的实现:
```java
public class Complex {
private double real;
private double imaginary;
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public double getReal() {
return real;
}
public double getImaginary() {
return imaginary;
}
public Complex add(Complex other) {
double newReal = this.real + other.real;
double newImaginary = this.imaginary + other.imaginary;
return new Complex(newReal, newImaginary);
}
public Complex subtract(Complex other) {
double newReal = this.real - other.real;
double newImaginary = this.imaginary - other.imaginary;
return new Complex(newReal, newImaginary);
}
public Complex multiply(Complex other) {
double newReal = this.real * other.real - this.imaginary * other.imaginary;
double newImaginary = this.real * other.imaginary + this.imaginary * other.real;
return new Complex(newReal, newImaginary);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imaginary * other.imaginary;
double newReal = (this.real * other.real + this.imaginary * other.imaginary) / denominator;
double newImaginary = (this.imaginary * other.real - this.real * other.imaginary) / denominator;
return new Complex(newReal, newImaginary);
}
@Override
public String toString() {
return String.format("%.2f + %.2fi", real, imaginary);
}
}
```
2. 复数运算实验
我们可以编写一些简单的测试来验证我们的复数类是否运行良好。例如,我们可以创建两个复数并将它们相加:
```java
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex result = c1.add(c2);
System.out.println(result);
}
```
输出结果应该为“4.00 + 6.00i”。
我们还可以测试减法、乘法和除法:
```java
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
Complex addResult = c1.add(c2);
Complex subtractResult = c1.subtract(c2);
Complex multiplyResult = c1.multiply(c2);
Complex divideResult = c1.divide(c2);
System.out.println("Addition: " + addResult);
System.out.println("Subtraction: " + subtractResult);
System.out.println("Multiplication: " + multiplyResult);
System.out.println("Division: " + divideResult);
}
```
输出结果应该为:
```
Addition: 4.00 + 6.00i
Subtraction: -2.00 - 2.00i
Multiplication: -5.00 + 10.00i
Division: 0.44 + 0.08i
```
3. 总结
我们已经成功地实现了一个Java复数类,并测试了它的基本数学运算。通过创建一个复数类,我们可以更轻松地进行复数计算,并且可以更容易地将复数与其他代码集成。
相关推荐
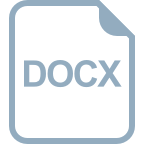

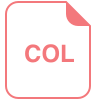
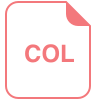
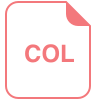
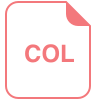









