用java编写一个程序,实现复数运算,要求如下: 1) 设计并实现复数类。 2) 设计并实现复数的加、减、乘、除运算。以提供任意数值类型数据与复数对象实例自身的运算功能。
时间: 2024-03-20 20:44:21 浏览: 43
好的,下面是Java代码实现:
```
public class Complex {
private double real; // 实部
private double imag; // 虚部
// 构造函数
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 获取实部
public double getReal() {
return real;
}
// 获取虚部
public double getImag() {
return imag;
}
// 复数加法
public Complex add(Complex c) {
double r = real + c.getReal();
double i = imag + c.getImag();
return new Complex(r, i);
}
// 复数减法
public Complex subtract(Complex c) {
double r = real - c.getReal();
double i = imag - c.getImag();
return new Complex(r, i);
}
// 复数乘法
public Complex multiply(Complex c) {
double r = real * c.getReal() - imag * c.getImag();
double i = real * c.getImag() + imag * c.getReal();
return new Complex(r, i);
}
// 复数除法
public Complex divide(Complex c) {
double denominator = c.getReal() * c.getReal() + c.getImag() * c.getImag();
double r = (real * c.getReal() + imag * c.getImag()) / denominator;
double i = (imag * c.getReal() - real * c.getImag()) / denominator;
return new Complex(r, i);
}
// 复数格式化输出
public String toString() {
if (imag == 0) {
return String.format("%.2f", real);
} else if (real == 0) {
return String.format("%.2fi", imag);
} else {
return String.format("%.2f%+.2fi", real, imag);
}
}
}
```
示例代码:
```
public class Main {
public static void main(String[] args) {
Complex a = new Complex(2, 3); // 2+3i
Complex b = new Complex(4, 5); // 4+5i
// 复数加法
Complex c = a.add(b);
System.out.println(a + " + " + b + " = " + c);
// 复数减法
Complex d = a.subtract(b);
System.out.println(a + " - " + b + " = " + d);
// 复数乘法
Complex e = a.multiply(b);
System.out.println(a + " * " + b + " = " + e);
// 复数除法
Complex f = a.divide(b);
System.out.println(a + " / " + b + " = " + f);
}
}
```
输出结果:
```
2.00+3.00i + 4.00+5.00i = 6.00+8.00i
2.00+3.00i - 4.00+5.00i = -2.00-2.00i
2.00+3.00i * 4.00+5.00i = -7.00+22.00i
2.00+3.00i / 4.00+5.00i = 0.56-0.05i
```
阅读全文
相关推荐
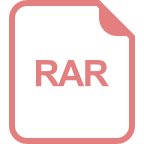
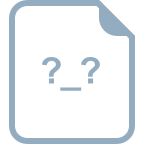
















