如何统计openmv框的个数代码
时间: 2023-08-03 08:04:02 浏览: 164
以下是OpenMV中统计矩形框个数的代码示例:
```
import sensor, image
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
sensor.set_auto_gain(False)
sensor.set_auto_whitebal(False)
thresholds = [(30, 100, -128, 127, -128, 127)]
roi = (0, 0, 320, 240)
while(True):
img = sensor.snapshot()
for r in img.find_rects(thresholds, roi=roi):
img.draw_rectangle(r.rect())
print("Number of rectangles: %d" % len(img.find_rects(thresholds, roi=roi)))
```
这个例子使用了 `find_rects()` 函数来找到矩形,并使用 `len()` 函数来计算找到的矩形数量。请注意,这个例子使用了阈值来查找矩形,并且使用了ROI来限制查找区域。你需要根据你的具体应用程序进行适当的修改。
相关问题
openmv统计像素点的个数
你可以使用OpenMV中的`image`模块中的`count_pixels()`函数来统计像素点的个数。该函数的语法如下:
```python
count = img.count_pixels(threshold=None, roi=None)
```
其中,`img`是你要统计像素点的图像,`threshold`是二值化的阈值,如果不传递该参数则会默认使用图像的中值作为阈值。`roi`是感兴趣区域的参数,如果不传递该参数则会统计整张图像中的像素点。
该函数会返回一个元组,其中包含了每个像素值的个数。例如,如果你要统计二值化图像中白色像素点的个数,可以使用以下代码:
```python
import sensor
import image
sensor.reset()
sensor.set_pixformat(sensor.GRAYSCALE)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time=2000)
img = sensor.snapshot().binary()
count = img.count_pixels(threshold=128)
print("White pixels: ", count[255])
```
在这个例子中,我们先抓取一张QVGA分辨率的灰度图像,然后进行二值化处理得到一个二值化图像,最后使用`count_pixels()`函数统计出二值化图像中白色像素点的个数。
用openmv识别图像中人脸的个数的相关代码
以下是使用OpenMV识别图像中人脸个数的示例代码:
```python
import sensor, image, time
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.GRAYSCALE)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
# 初始化Haar级联分类器
face_cascade = image.HaarCascade("frontalface", stages=25)
print(face_cascade)
while True:
img = sensor.snapshot()
# 检测人脸
objects = img.find_features(face_cascade, threshold=0.5, scale_factor=1.5)
# 绘制检测框
for r in objects:
img.draw_rectangle(r)
print("Detected %d faces." % len(objects))
```
该代码中通过使用Haar级联分类器进行人脸检测,具体操作步骤如下:
1. 初始化摄像头。
2. 初始化Haar级联分类器,使用预训练好的“frontalface”分类器。
3. 进入循环,拍摄一张图片。
4. 使用`find_features()`函数检测人脸,返回的是一个包含所有检测框坐标的列表。
5. 遍历列表,使用`draw_rectangle()`函数绘制检测框。
6. 打印检测到的人脸个数。
需要注意的是,该代码中的`threshold`参数和`scale_factor`参数都可以调整,以达到更好的检测效果。另外,该代码只能检测正面的人脸,对于侧面或者其他角度的人脸可能无法检测到。
阅读全文
相关推荐
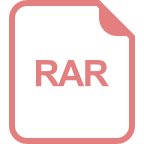
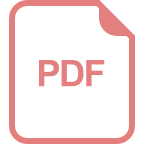
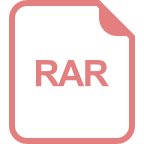
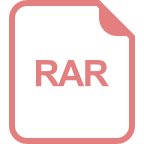
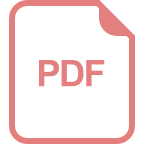
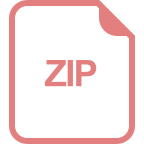
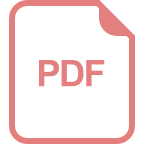
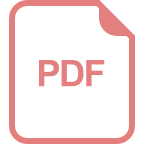
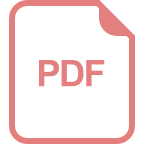
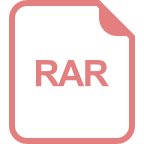
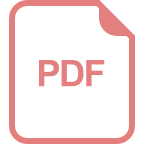


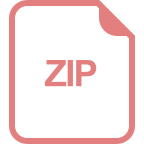
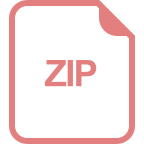