sort(alls.begin(), alls.end());
时间: 2024-05-22 11:13:43 浏览: 98
This line of code sorts the elements in the vector called "alls" in ascending order. The function "sort" is part of the C++ standard library and takes two arguments: the first is an iterator to the beginning of the range to be sorted, and the second is an iterator to the end of the range to be sorted. In this case, "alls.begin()" returns an iterator to the first element of the vector and "alls.end()" returns an iterator to one past the last element of the vector. Therefore, the entire vector is sorted. The elements in the vector must be comparable using the less-than operator (operator<), which is used by default.
相关问题
#include <iostream> #include <vector> #include <algorithm> using namespace std; typedef pair<int,int> PII; const int N = 300010; int n, m; int a[N]; //每项 int s[N]; //前缀和 vector <int> alls; vector <PII> add,query; //PII是一个包含两个int成员变量的结构体 int find(int x) { int l = 0, r = alls.size() - 1; while (l < r) { int mid = l + r >> 1; if (alls[mid] >= x) r = mid; else l = mid + 1; } return r + 1; } int main() { cin >> n >> m; for(int i = 0; i < 0; i ++ ) { int x, c; cin >> x >> c; add.push_back({x, c}); alls.push_back(x); } for(int i = 0; i < m; i ++ ) { int l, r; cin >> l >> r; query.push_back({l, r}); alls.push_back(l); alls.push_back(r); } sort(alls.begin(), alls.end()); //排序 alls.erase(unique(alls.begin(), alls.end()), alls.end()); // 去重 // 处理插入 for (auto item : add) { int x = find(item.first); a[x] += item.second; } //"for(auto item:add)" 是一种 C++11 的语法,它表示使用 C++11 的新特性 "Range-based for loop", //用于遍历容器中的所有元素。其中 "add" 是一个容器,"item" 是一个变量,每次循环都会将容器中的下一个元素赋值给该变量。 // 预处理前缀和 for (int i = 1; i <= alls.size(); i ++ ) s[i] = s[i - 1] + a[i]; // 处理询问 for (auto item : query) { int l = find(item.first), r = find(item.second); cout << s[r] - s[l - 1] << endl; } return 0; }
这段代码是一个求前缀和的例子,它包含了使用 "for(auto item:add)" 和 "for(auto item:query)" 的用法。
首先,代码定义了一个名为 "add" 的 vector,其中存储了一组 pair<int,int> 类型的数据,表示要添加的元素。它还定义了一个名为 "query" 的 vector,其中存储了一组 pair<int,int> 类型的数据,表示查询的区间。
然后,代码使用 "for(auto item : add)" 遍历了 "add" 容器中的每个元素。每次循环,都会将容器中的下一个元素赋值给变量 "item"。在代码中,使用了结构化绑定语法将 pair<int,int> 类型的元素分别绑定到变量 "x" 和 "c",然后根据需求进行处理。
接着,代码使用 "for(auto item : query)" 遍历了 "query" 容器中的每个元素。同样地,每次循环都会将容器中的下一个元素赋值给变量 "item"。在代码中,使用了结构化绑定语法将 pair<int,int> 类型的元素分别绑定到变量 "l" 和 "r",然后根据需求进行处理。
总的来说,这段代码利用了 C++11 中的 Range-based for loop 语法,简化了遍历容器的过程,并且可以方便地访问容器中的元素。
忽略该脚本警告 import pandas as pd import glob def com(): file_paths = glob.glob('E:/py卓望/数据分析/top150_20230321/*.txt') data = pd.DataFrame() for i in file_paths: df = pd.read_csv(i, sep=',', header=None, skiprows=[0]) data = pd.concat([data, df]) data.drop(df.columns[0], axis=1, inplace=True) df.sort_values(by=1, ascending=False, inplace=True) data.iloc[:, 0] = data.iloc[:, 0].str.lower() data.to_csv('E:/py卓望/数据分析/all/all_file.txt', sep=',', index=False,header=False) all = pd.read_csv('E:/py卓望/数据分析/all/all_file.txt', header=None, delimiter=',') all[0] = all[0].str.split('.') all[0] = all[0].apply( lambda x: '.'.join(x[-3:]) if '.'.join(x[-2:]) in ['gov.cn', 'com.cn', 'org.cn', 'net.cn'] else '.'.join(x[-2:])) new_col = all[0] result = pd.concat([new_col,all.iloc[:,1:]],axis=1) result.to_csv('E:/py卓望/数据分析/all/二级域名.txt', sep=',',index=False,header=False) summation = pd.read_csv('E:/py卓望/数据分析/all/二级域名.txt', header=None, delimiter=',') grouped = summation.groupby(0)[1].sum().reset_index() grouped = grouped.sort_values(by=1, ascending=False).reset_index(drop=True) grouped[1] = grouped[1].fillna(summation[1]) grouped.to_csv('E:/py卓望/数据分析/all/处理后求和域名.txt', sep=',', index=False, header=False) top_10000 = pd.read_csv('E:/py卓望/数据分析/all/处理后求和域名.txt', header=None, delimiter=',') alls = top_10000.nlargest(10000, 1) alls.drop(columns=[1], inplace=True) alls.to_csv('E:/py卓望/数据分析/all/data.txt', sep=',',index=False, header=False) final = top_10000.iloc[10000:] final.drop(columns=[1], inplace=True) final.to_csv('E:/py卓望/数据分析/all/final_data.txt', sep=',',index=False, header=False) print(final.to_csv) warnings.filterwarnings("ignore") def main(): com() if __name__ == "__main__": print("开始清洗域名文件") main() print("数据清洗完毕")
要忽略该脚本中的警告,可以在脚本开头添加以下两行代码:
```python
import warnings
warnings.filterwarnings("ignore")
```
这会忽略脚本中的所有警告。将这两行代码添加到脚本的开头即可:
```python
import pandas as pd
import glob
import warnings
def com():
warnings.filterwarnings("ignore")
file_paths = glob.glob('E:/py卓望/数据分析/top150_20230321/*.txt')
data = pd.DataFrame()
for i in file_paths:
df = pd.read_csv(i, sep=',', header=None, skiprows=[0])
data = pd.concat([data, df])
data.drop(df.columns[0], axis=1, inplace=True)
df.sort_values(by=1, ascending=False, inplace=True)
data.iloc[:, 0] = data.iloc[:, 0].str.lower()
data.to_csv('E:/py卓望/数据分析/all/all_file.txt', sep=',', index=False,header=False)
all = pd.read_csv('E:/py卓望/数据分析/all/all_file.txt', header=None, delimiter=',')
all[0] = all[0].str.split('.')
all[0] = all[0].apply(
lambda x: '.'.join(x[-3:]) if '.'.join(x[-2:]) in ['gov.cn', 'com.cn', 'org.cn', 'net.cn'] else '.'.join(x[-2:]))
new_col = all[0]
result = pd.concat([new_col,all.iloc[:,1:]],axis=1)
result.to_csv('E:/py卓望/数据分析/all/二级域名.txt', sep=',',index=False,header=False)
summation = pd.read_csv('E:/py卓望/数据分析/all/二级域名.txt', header=None, delimiter=',')
grouped = summation.groupby(0)[1].sum().reset_index()
grouped = grouped.sort_values(by=1, ascending=False).reset_index(drop=True)
grouped[1] = grouped[1].fillna(summation[1])
grouped.to_csv('E:/py卓望/数据分析/all/处理后求和域名.txt', sep=',', index=False, header=False)
top_10000 = pd.read_csv('E:/py卓望/数据分析/all/处理后求和域名.txt', header=None, delimiter=',')
alls = top_10000.nlargest(10000, 1)
alls.drop(columns=[1], inplace=True)
alls.to_csv('E:/py卓望/数据分析/all/data.txt', sep=',',index=False, header=False)
final = top_10000.iloc[10000:]
final.drop(columns=[1], inplace=True)
final.to_csv('E:/py卓望/数据分析/all/final_data.txt', sep=',',index=False, header=False)
print(final.to_csv)
def main():
print("开始清洗域名文件")
com()
print("数据清洗完毕")
if __name__ == "__main__":
main()
```
这样就可以在运行脚本时忽略所有警告了。
阅读全文
相关推荐
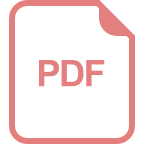
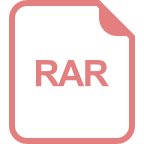
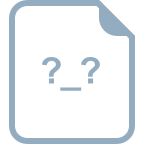
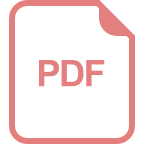
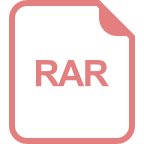
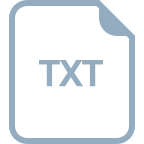
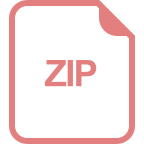
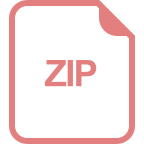
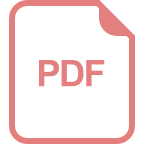
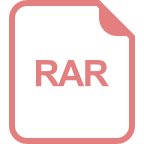

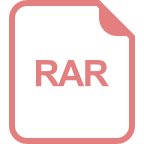
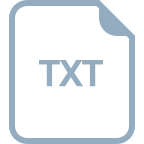
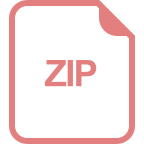