请写一个可以添加、显示、查找、删除、保存、导出学生成绩记录的学生成绩管理系统c++
时间: 2023-07-10 12:33:26 浏览: 84
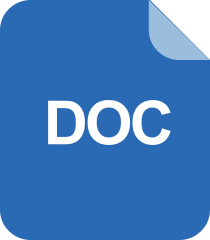
用C++编写一个学生成绩管理系统

好的,我可以为你提供一个简单的学生成绩管理系统的C++代码,包含添加、显示、查找、删除、保存、导出学生成绩记录等功能。以下是代码实现:
```c++
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
#include <vector>
using namespace std;
// 学生类
class Student {
public:
string name; // 姓名
string id; // 学号
double score; // 成绩
Student(string name = "", string id = "", double score = 0.0) {
this->name = name;
this->id = id;
this->score = score;
}
};
// 学生成绩管理类
class GradeManager {
private:
vector<Student> students; // 学生记录
// 内部函数:查找学生索引位置
int findIndex(string id) {
for (int i = 0; i < students.size(); i++) {
if (students[i].id == id) {
return i;
}
}
return -1;
}
public:
// 添加学生记录
void addStudent(Student student) {
students.push_back(student);
}
// 显示所有学生记录
void showAllStudents() {
cout << "姓名\t学号\t成绩" << endl;
for (int i = 0; i < students.size(); i++) {
cout << students[i].name << "\t" << students[i].id << "\t" << fixed << setprecision(2) << students[i].score << endl;
}
}
// 查找学生记录
void searchStudent(string id) {
int index = findIndex(id);
if (index >= 0) {
cout << "找到学生:" << students[index].name << "\t" << students[index].id << "\t" << fixed << setprecision(2) << students[index].score << endl;
} else {
cout << "未找到该学生" << endl;
}
}
// 删除学生记录
void deleteStudent(string id) {
int index = findIndex(id);
if (index >= 0) {
students.erase(students.begin() + index);
cout << "删除成功" << endl;
} else {
cout << "未找到该学生" << endl;
}
}
// 保存学生成绩记录到文件
void saveToFile(string filename) {
ofstream outfile(filename);
if (outfile.is_open()) {
for (int i = 0; i < students.size(); i++) {
outfile << students[i].name << "\t" << students[i].id << "\t" << fixed << setprecision(2) << students[i].score << endl;
}
outfile.close();
cout << "保存成功" << endl;
} else {
cout << "保存失败" << endl;
}
}
// 从文件中导入学生成绩记录
void loadFromFile(string filename) {
ifstream infile(filename);
if (infile.is_open()) {
students.clear();
string line;
while (getline(infile, line)) {
string name, id;
double score;
sscanf(line.c_str(), "%s%s%lf", &name, &id, &score);
Student student(name, id, score);
students.push_back(student);
}
infile.close();
cout << "导入成功" << endl;
} else {
cout << "导入失败" << endl;
}
}
// 导出学生成绩记录到文件
void exportToFile(string filename) {
ofstream outfile(filename);
if (outfile.is_open()) {
outfile << "姓名\t学号\t成绩" << endl;
for (int i = 0; i < students.size(); i++) {
outfile << students[i].name << "\t" << students[i].id << "\t" << fixed << setprecision(2) << students[i].score << endl;
}
outfile.close();
cout << "导出成功" << endl;
} else {
cout << "导出失败" << endl;
}
}
};
// 主函数
int main() {
GradeManager manager;
while (true) {
cout << "请输入操作序号:" << endl;
cout << "1. 添加学生记录" << endl;
cout << "2. 显示所有学生记录" << endl;
cout << "3. 查找学生记录" << endl;
cout << "4. 删除学生记录" << endl;
cout << "5. 保存学生成绩记录到文件" << endl;
cout << "6. 从文件中导入学生成绩记录" << endl;
cout << "7. 导出学生成绩记录到文件" << endl;
cout << "0. 退出程序" << endl;
int choice;
cin >> choice;
if (choice == 1) {
cout << "请输入学生姓名、学号、成绩:" << endl;
string name, id;
double score;
cin >> name >> id >> score;
Student student(name, id, score);
manager.addStudent(student);
cout << "添加成功" << endl;
} else if (choice == 2) {
manager.showAllStudents();
} else if (choice == 3) {
cout << "请输入要查找的学生学号:" << endl;
string id;
cin >> id;
manager.searchStudent(id);
} else if (choice == 4) {
cout << "请输入要删除的学生学号:" << endl;
string id;
cin >> id;
manager.deleteStudent(id);
} else if (choice == 5) {
cout << "请输入要保存学生成绩记录的文件名:" << endl;
string filename;
cin >> filename;
manager.saveToFile(filename);
} else if (choice == 6) {
cout << "请输入要导入学生成绩记录的文件名:" << endl;
string filename;
cin >> filename;
manager.loadFromFile(filename);
} else if (choice == 7) {
cout << "请输入要导出学生成绩记录的文件名:" << endl;
string filename;
cin >> filename;
manager.exportToFile(filename);
} else if (choice == 0) {
break;
} else {
cout << "输入错误,请重新输入" << endl;
}
}
return 0;
}
```
这是一个简单的学生成绩管理系统,其中包含了添加、显示、查找、删除、保存、导出学生成绩记录等功能。你可以根据自己的需求进行修改和完善。
阅读全文
相关推荐










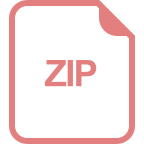
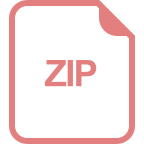
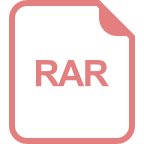
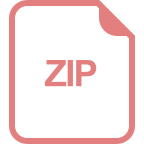