基于Java的GUI日历记事本应用程序
时间: 2023-07-26 14:45:03 浏览: 71
好的,这里是一个简单的基于Java的GUI日历记事本应用程序的实现思路:
1. GUI界面设计
设计一个主窗口,包含一个日历控件和一个文本框。当用户选择一个日期时,程序将会自动加载对应的日志内容到文本框中。当用户输入日志内容时,程序将会自动保存到对应的日期中。
2. 数据结构设计
使用一个HashMap来保存每个日期对应的日志内容,日期作为键,日志内容作为值。这样可以快速地根据日期查找对应的日志内容。同时,可以使用文件来持久化保存数据,这样即使程序关闭,数据也能够得以保存。
3. 算法和优化
为了提高时间和空间效率,可以考虑使用一些常见的算法和数据结构,比如哈希表、二分查找、红黑树等。同时,需要注意内存和文件读写的优化,避免出现性能瓶颈。
4. 代码实现
在代码实现中,需要使用Swing或JavaFX等工具来实现GUI界面,以及使用Java的IO操作来进行文件读写。具体实现细节需要根据具体需求来进行调整。
下面是一个示例代码,实现了一个基本的GUI日历记事本应用程序:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.text.SimpleDateFormat;
import java.util.*;
public class CalendarNote extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
private static final String DATA_FILE = "notes.csv";
private static final String[] WEEKDAYS = new String[]{"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"};
private static final int WINDOW_WIDTH = 600;
private static final int WINDOW_HEIGHT = 400;
private static final int CALENDAR_WIDTH = 400;
private static final int CALENDAR_HEIGHT = 250;
private static final int NOTE_WIDTH = 400;
private static final int NOTE_HEIGHT = 150;
private static final String NEW_LINE = System.getProperty("line.separator");
private Map<String, String> notes = new HashMap<>();
private JTextArea noteArea = new JTextArea(NOTE_HEIGHT / 20, NOTE_WIDTH / 20);
private JPanel calendar = new JPanel(new GridLayout(7, 7));
private JLabel monthYearLabel = new JLabel();
private Calendar currentCalendar = Calendar.getInstance();
public CalendarNote() {
setTitle("Calendar Note");
setSize(WINDOW_WIDTH, WINDOW_HEIGHT);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
initGUI();
loadNotes();
updateNoteArea(currentCalendar.getTime());
}
private void initGUI() {
JPanel mainPanel = new JPanel(new BorderLayout());
mainPanel.add(createCalendarPanel(), BorderLayout.WEST);
mainPanel.add(createNotePanel(), BorderLayout.CENTER);
setContentPane(mainPanel);
}
private JPanel createCalendarPanel() {
JPanel panel = new JPanel(new BorderLayout());
calendar.setPreferredSize(new Dimension(CALENDAR_WIDTH, CALENDAR_HEIGHT));
panel.add(createMonthYearPanel(), BorderLayout.NORTH);
panel.add(calendar, BorderLayout.CENTER);
updateCalendar(currentCalendar);
return panel;
}
private JPanel createNotePanel() {
JPanel panel = new JPanel(new BorderLayout());
noteArea.setLineWrap(true);
JScrollPane scrollPane = new JScrollPane(noteArea);
scrollPane.setPreferredSize(new Dimension(NOTE_WIDTH, NOTE_HEIGHT));
panel.add(scrollPane, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new FlowLayout(FlowLayout.RIGHT));
JButton saveButton = new JButton("Save");
saveButton.addActionListener(this);
buttonPanel.add(saveButton);
panel.add(buttonPanel, BorderLayout.SOUTH);
return panel;
}
private JPanel createMonthYearPanel() {
JPanel panel = new JPanel(new FlowLayout());
JButton previousButton = new JButton("<");
JButton nextButton = new JButton(">");
previousButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
currentCalendar.add(Calendar.MONTH, -1);
updateCalendar(currentCalendar);
}
});
nextButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
currentCalendar.add(Calendar.MONTH, 1);
updateCalendar(currentCalendar);
}
});
panel.add(previousButton);
panel.add(monthYearLabel);
panel.add(nextButton);
return panel;
}
private void updateCalendar(Calendar calendar) {
calendar.set(Calendar.DATE, 1);
int firstDayOfWeek = calendar.get(Calendar.DAY_OF_WEEK);
int daysInMonth = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
calendar.add(Calendar.MONTH, -1);
int daysInPreviousMonth = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
calendar.add(Calendar.MONTH, 1);
monthYearLabel.setText((calendar.get(Calendar.MONTH) + 1) + "/" + calendar.get(Calendar.YEAR));
calendar.removeAll();
for (int i = 0; i < WEEKDAYS.length; i++) {
JLabel label = new JLabel(WEEKDAYS[i], JLabel.CENTER);
calendar.add(label);
}
int day = 2 - firstDayOfWeek;
if (day > 1) {
day -= 7;
}
for (int i = 0; i < 42; i++) {
if (i % 7 == 0) {
calendar.add(new JLabel(""));
} else {
if (day < 1) {
JButton button = new JButton("" + (day + daysInPreviousMonth));
button.setForeground(Color.GRAY);
calendar.add(button);
} else if (day > daysInMonth) {
JButton button = new JButton("" + (day - daysInMonth));
button.setForeground(Color.GRAY);
calendar.add(button);
} else {
JButton button = new JButton("" + day);
String dateStr = calendar.get(Calendar.YEAR) + "-" + (calendar.get(Calendar.MONTH) + 1) + "-" + day;
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateNoteArea(parseDate(dateStr));
}
});
calendar.add(button);
}
day++;
}
}
}
private void loadNotes() {
File file = new File(DATA_FILE);
if (file.exists()) {
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(",");
if (fields.length == 2) {
notes.put(fields[0], fields[1]);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
private void saveNotes() {
try (PrintWriter writer = new PrintWriter(new FileWriter(DATA_FILE))) {
for (Map.Entry<String, String> entry : notes.entrySet()) {
writer.println(entry.getKey() + "," + entry.getValue());
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void updateNoteArea(Date date) {
String note = notes.get(dateFormat.format(date));
noteArea.setText(note != null ? note : "");
}
private void saveNote() {
String note = noteArea.getText();
notes.put(dateFormat.format(currentCalendar.getTime()), note);
saveNotes();
}
private Date parseDate(String dateStr) {
try {
return dateFormat.parse(dateStr);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public void actionPerformed(ActionEvent e) {
saveNote();
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new CalendarNote().setVisible(true);
}
});
}
}
```
这个程序使用了Swing库来实现GUI界面,使用了HashMap来存储每个日期对应的日志内容,使用了文件来进行数据持久化。程序中还包含了读取和保存数据的操作。程序启动时会自动加载数据,当用户选择一个日期时,程序会自动加载对应的日志内容到文本框中,当用户输入日志内容时,程序会自动保存到对应的日期中。
相关推荐
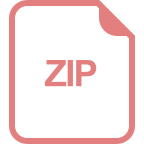














