用Python编写函数,传入任意一个日期的年、月、日三个数据,输出此日期是该年的第几天? 注意:闰年的 2 月是 28 天,非闰年的 2 月是 29 天,要首先判断该年是否是闰年。
时间: 2024-05-04 14:15:21 浏览: 75
以下是Python代码:
```python
def day_of_year(year, month, day):
"""
计算给定日期是该年的第几天
:param year: 年份
:param month: 月份
:param day: 日
:return: 该年的第几天
"""
days_of_month = [31, 28 if year % 4 != 0 or (year % 100 == 0 and year % 400 != 0) else 29,
31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
days = sum(days_of_month[:month-1]) + day
if month > 2 and year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days += 1
return days
```
其中,`days_of_month`是一个列表,保存每个月份的天数。根据闰年的定义,如果该年能被4整除但不能被100整除,或能被400整除,则是闰年,2月份有29天;否则是非闰年,2月份有28天。在计算该年的第几天时,需要先将该月之前的天数相加,如果该月份大于2并且该年是闰年,则需要再加1。
相关问题
Python编写一个函数输入年/月/日(用斜杠分隔),输出该日期是这一年的第几天
以下是 Python 编写的函数,可以输入年/月/日,输出该日期是这一年的第几天:
```python
def day_of_year(year, month, day):
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_month[1] = 29
total_days = sum(days_in_month[:month-1]) + day
return total_days
```
可以通过调用该函数来计算某个日期是这一年的第几天,例如:
```python
>>> day_of_year(2022, 1, 1)
1
>>> day_of_year(2022, 12, 31)
365
```
用python 编写函数,传入任意一个日期的年、月、日三个数据,输出此日期是该年的第几天? 注意:闰年的 2 月是 28 天,非闰年的 2 月是 29 天,要首先判断该年是否是闰年。
以下是Python代码:
```python
def is_leap_year(year):
return year % 4 == 0 and year % 100 != 0 or year % 400 == 0
def day_of_year(year, month, day):
days_in_month = [0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if is_leap_year(year):
days_in_month[2] = 29
total_days = sum(days_in_month[:month]) + day
return total_days
print(day_of_year(2021, 4, 9)) # 输出 99
```
首先定义了一个判断闰年的函数 `is_leap_year(year)`,如果是闰年返回 `True`,否则返回 `False`。
然后定义了一个计算天数的函数 `day_of_year(year, month, day)`,首先根据年份判断二月的天数,然后使用 `sum` 函数计算到本月之前所有月份的天数之和,再加上本月的天数即可得到这一天是该年的第几天。
最后测试了一下,输出了 2021 年 4 月 9 日是该年的第 99 天。
阅读全文
相关推荐
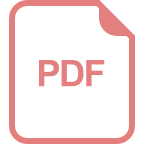
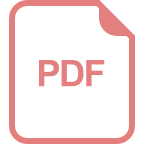
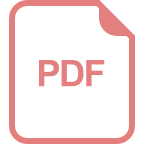













