详情页点击加入购物车,商品出现在购物车页面如何实现
时间: 2024-04-01 09:37:39 浏览: 18
要实现商品详情页点击加入购物车后,商品出现在购物车页面,您需要进行以下几个步骤:
1. 在商品详情页中,添加一个表单,其中包含一个隐藏的产品ID字段和一个数量字段,还有一个提交按钮。当用户点击提交按钮时,表单数据将被提交到一个处理程序中,该处理程序将产品ID和数量添加到购物车中。
2. 在处理程序中,将产品ID和数量添加到购物车中。购物车可以是一个数组、一个数据库表或其他数据结构,具体实现方式取决于您的需求。
3. 在购物车页面中,通过循环遍历购物车中的所有商品,将它们显示出来。您可以使用HTML和CSS来创建购物车页面,或者使用一个购物车模板库来快速创建。
以下是一个简单的示例代码,用于向购物车中添加商品并显示购物车页面:
add_to_cart.php
```php
<?php
session_start();
// 获取产品ID和数量
$product_id = $_POST['product_id'];
$quantity = $_POST['quantity'];
// 将产品添加到购物车中
if (!isset($_SESSION['cart'])) {
$_SESSION['cart'] = array();
}
if (isset($_SESSION['cart'][$product_id])) {
$_SESSION['cart'][$product_id] += $quantity;
} else {
$_SESSION['cart'][$product_id] = $quantity;
}
```
cart.php
```php
<?php
session_start();
// 获取购物车中的所有商品
$cart_items = array();
if (isset($_SESSION['cart'])) {
foreach ($_SESSION['cart'] as $product_id => $quantity) {
// 从数据库中获取产品信息
$product = get_product_by_id($product_id);
// 计算产品总价
$total_price = $product['price'] * $quantity;
// 将产品添加到购物车项目数组中
$cart_items[] = array(
'product_id' => $product_id,
'product_name' => $product['name'],
'product_image' => $product['image'],
'product_price' => $product['price'],
'quantity' => $quantity,
'total_price' => $total_price
);
}
}
// 显示购物车页面
include 'cart_template.php';
```
cart_template.php
```html
<!DOCTYPE html>
<html>
<head>
<title>购物车</title>
</head>
<body>
<h1>购物车</h1>
<?php if (count($cart_items) == 0): ?>
<p>购物车为空</p>
<?php else: ?>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>数量</th>
<th>单价</th>
<th>总价</th>
</tr>
</thead>
<tbody>
<?php foreach ($cart_items as $item): ?>
<tr>
<td><?php echo $item['product_name']; ?></td>
<td><?php echo $item['quantity']; ?></td>
<td><?php echo $item['product_price']; ?></td>
<td><?php echo $item['total_price']; ?></td>
</tr>
<?php endforeach; ?>
</tbody>
</table>
<?php endif; ?>
</body>
</html>
```
在上述示例中,我们使用了一个名为`$_SESSION`的超全局变量来保存购物车数据,这是PHP中一种方便的保存会话数据的方式。请注意,这只是一个简单的示例,实际中您需要根据自己的需求进行更详细的实现。
相关推荐
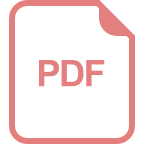
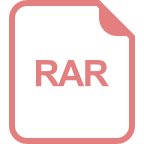















