使用pyqt编写随鼠标移动的放大镜
时间: 2023-07-05 10:08:39 浏览: 49
以下是使用 PyQt 编写的随鼠标移动的放大镜示例:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout
from PyQt5.QtGui import QPixmap, QCursor, QPainter, QPen, QBrush
from PyQt5.QtCore import Qt, QPoint
class MagnifyingGlass(QWidget):
def __init__(self):
super().__init__()
self.setWindowFlags(Qt.FramelessWindowHint | Qt.WindowStaysOnTopHint | Qt.Tool)
self.setAttribute(Qt.WA_TranslucentBackground)
self.label = QLabel(self)
self.label.setScaledContents(True)
self.pix = QPixmap('image.jpg')
self.label.setPixmap(self.pix)
self.magnify = 2.0
self.resize(self.pix.width() // self.magnify, self.pix.height() // self.magnify)
self.setMouseTracking(True)
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
painter.setPen(QPen(Qt.red, 1))
painter.setBrush(QBrush(Qt.red, Qt.SolidPattern))
painter.drawEllipse(self.width() // 2 - 5, self.height() // 2 - 5, 10, 10)
def mouseMoveEvent(self, event):
cursor_pos = QCursor.pos()
x = cursor_pos.x() - self.width() // 2
y = cursor_pos.y() - self.height() // 2
if x < 0:
x = 0
elif x > self.pix.width() - self.width() // self.magnify:
x = self.pix.width() - self.width() // self.magnify
if y < 0:
y = 0
elif y > self.pix.height() - self.height() // self.magnify:
y = self.pix.height() - self.height() // self.magnify
self.label.setPixmap(self.pix.copy(x * self.magnify, y * self.magnify, self.width() * self.magnify, self.height() * self.magnify))
self.move(cursor_pos.x() - self.width() // 2, cursor_pos.y() - self.height() // 2)
def resizeEvent(self, event):
self.label.resize(self.size())
if __name__ == '__main__':
app = QApplication(sys.argv)
magnify = MagnifyingGlass()
magnify.show()
sys.exit(app.exec_())
```
该示例中,我们使用了 `QLabel` 来显示要放大的图片,使用 `QPainter` 绘制了放大镜的圆形边框,并且使用 `setMouseTracking(True)` 方法来跟踪鼠标的移动。当鼠标移动时,我们获取到鼠标的位置 `cursor_pos`,然后根据放大倍数 `magnify` 计算出要显示的区域,并将其设置为 `QLabel` 的 pixmap。最后,使用 `move()` 方法将放大镜移动到鼠标所在的位置。
相关推荐
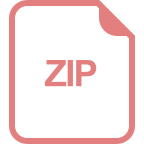
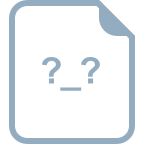














