MFC unicode DWORD转换 CString
时间: 2024-01-09 07:03:47 浏览: 171
MFC中可以使用CString的Format函数将DWORD转换为一个Unicode字符串,例如:
```cpp
DWORD dwValue = 123456;
CString strValue;
strValue.Format(L"%u", dwValue);
```
在这个例子中,L前缀表示字符串是Unicode格式的。%u表示将无符号整数输出为十进制数。将这个无符号整数dwValue传递给Format函数,它将返回一个Unicode格式的CString对象strValue,其中包含转换后的字符串值。
相关问题
MFC UNICODE int 转CString
你可以使用CString的Format方法将整数转换为CString,如下所示:
```cpp
int num = 123;
CString str;
str.Format(L"%d", num);
```
其中,`%d`是格式化字符串中的占位符,用于表示整数类型。`L`前缀表示使用Unicode编码。如果你的MFC项目中没有启用Unicode,可以将`L`前缀去掉。
MFC UNICODE下 CByteArray 转为CString
在MFC UNICODE下,可以使用CString的Format函数和CByteArray的GetData函数,将CByteArray转换为CString。具体代码如下:
```c++
CByteArray byteArray; // 假设有一个CByteArray对象
byteArray.Add(0x41); // 向byteArray中添加一个字节数据
byteArray.Add(0x42); // 向byteArray中添加另一个字节数据
CString str;
str.Format(_T("%s"), (LPCTSTR)CA2W((char*)byteArray.GetData())); // 将CByteArray转换为char*,再转换为LPCTSTR,并赋值给CString对象
```
上述代码使用CA2W将CByteArray转换为char*,再将char*转换为LPCTSTR,并使用Format赋值给CString对象,从而将CByteArray转换为CString。
阅读全文
相关推荐
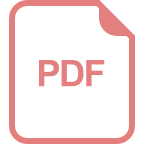
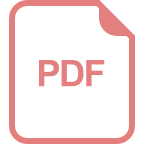
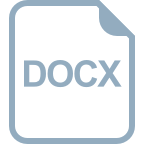













