python先序遍历和中序遍历求二叉树
时间: 2023-09-08 11:08:18 浏览: 50
可以通过先序遍历和中序遍历的结果重构二叉树。具体步骤如下:
1. 先序遍历的第一个元素是根节点,找到该元素在中序遍历中的位置,划分出左右子树的中序遍历序列。
2. 根据左右子树的中序遍历序列长度,划分出左右子树的先序遍历序列。
3. 递归构建左右子树。
Python代码实现如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def buildTree(preorder: List[int], inorder: List[int]) -> TreeNode:
if not preorder or not inorder:
return None
root_val = preorder[0]
root = TreeNode(root_val)
root_index = inorder.index(root_val)
left_inorder = inorder[:root_index]
right_inorder = inorder[root_index+1:]
left_preorder = preorder[1:1+len(left_inorder)]
right_preorder = preorder[len(left_inorder)+1:]
root.left = buildTree(left_preorder, left_inorder)
root.right = buildTree(right_preorder, right_inorder)
return root
```
其中,preorder和inorder分别为先序遍历和中序遍历的结果,返回重构后的二叉树的根节点。
相关推荐
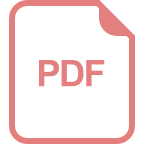
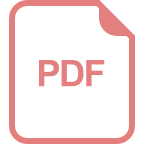
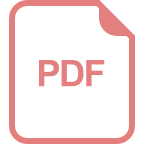














