用单层感知器实现一个mnist手写数字识别
时间: 2023-11-12 12:05:11 浏览: 44
单层感知器是一种简单的神经网络模型,它可以用来解决二分类问题。对于手写数字识别问题,我们需要将其转化为一个多分类问题,因此单层感知器无法满足我们的需求。我们可以使用多层感知器(MLP)来解决这个问题。
以下是使用MLP实现MNIST手写数字识别的Python代码:
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
# 加载MNIST数据集
mnist = input_data.read_data_sets('MNIST_data', one_hot=True)
# 定义输入和输出的占位符
x = tf.placeholder(tf.float32, [None, 784])
y_ = tf.placeholder(tf.float32, [None, 10])
# 定义隐藏层和输出层
W1 = tf.Variable(tf.random_normal([784, 256]))
b1 = tf.Variable(tf.zeros([256]))
hidden1 = tf.nn.relu(tf.matmul(x, W1) + b1)
W2 = tf.Variable(tf.random_normal([256, 10]))
b2 = tf.Variable(tf.zeros([10]))
y = tf.matmul(hidden1, W2) + b2
# 定义损失函数和优化器
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y_, logits=y))
train_step = tf.train.AdamOptimizer(1e-4).minimize(cross_entropy)
# 定义准确率
correct_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 开始训练模型
sess = tf.InteractiveSession()
tf.global_variables_initializer().run()
for i in range(10000):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y_: batch_ys})
if i % 1000 == 0:
train_accuracy = accuracy.eval(feed_dict={x: batch_xs, y_: batch_ys})
print('step %d, training accuracy %g' % (i, train_accuracy))
# 在测试集上测试准确率
print('test accuracy %g' % accuracy.eval(feed_dict={x: mnist.test.images, y_: mnist.test.labels}))
```
在这个代码中,我们使用了一个两层的MLP来解决MNIST手写数字识别问题。第一层是有256个神经元的隐藏层,第二层是有10个神经元的输出层(对应于10个数字)。我们使用ReLU激活函数来激活隐藏层,并使用softmax交叉熵作为损失函数。我们使用Adam优化器来优化模型。在训练模型之后,我们在测试集上测试了模型的准确率。
相关推荐
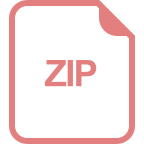
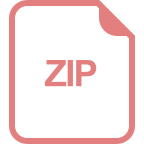
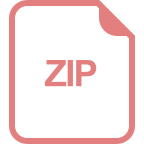
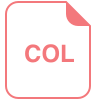













