c语言中怎么获取视频帧数
时间: 2023-05-27 19:02:57 浏览: 215
获取视频帧数需要使用视频处理库,比如FFmpeg。以下是使用FFmpeg获取视频帧数的示例代码:
```c
#include <stdio.h>
#include <libavformat/avformat.h>
int main(int argc, char **argv)
{
AVFormatContext *pFormatCtx = NULL;
int i, videoStream;
AVRational videoTimeBase;
AVCodecParameters *pCodecPar = NULL;
int64_t duration, totalFrames;
if (argc < 2) {
printf("Usage: %s <video_file>\n", argv[0]);
return -1;
}
// Open video file
if (avformat_open_input(&pFormatCtx, argv[1], NULL, NULL) != 0) {
printf("Error: Could not open file '%s'\n", argv[1]);
return -1;
}
// Retrieve stream information
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
printf("Error: Could not find stream information\n");
return -1;
}
// Dump information about file onto standard error
av_dump_format(pFormatCtx, 0, argv[1], 0);
// Find the first video stream
videoStream = -1;
for (i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStream = i;
break;
}
}
if (videoStream == -1) {
printf("Error: Could not find video stream\n");
return -1;
}
// Get video parameters
pCodecPar = pFormatCtx->streams[videoStream]->codecpar;
videoTimeBase = pFormatCtx->streams[videoStream]->time_base;
// Calculate video duration and total frames
duration = pFormatCtx->duration;
totalFrames = (duration * pCodecPar->frame_rate.num + pCodecPar->frame_rate.den - 1) / pCodecPar->frame_rate.den;
printf("File '%s':\n", argv[1]);
printf(" Duration: %" PRId64 " seconds\n", duration / AV_TIME_BASE);
printf(" Video stream:\n");
printf(" Codec: %s\n", avcodec_get_name(pCodecPar->codec_id));
printf(" Resolution: %dx%d\n", pCodecPar->width, pCodecPar->height);
printf(" Frame rate: %.2f\n", (double)pCodecPar->frame_rate.num / pCodecPar->frame_rate.den);
printf(" Total frames: %" PRId64 "\n", totalFrames);
// Close video file
avformat_close_input(&pFormatCtx);
return 0;
}
```
编译代码需要链接FFmpeg库,比如使用以下命令:
```
gcc -o get_frames get_frames.c -lavformat
```
运行程序需要传递视频文件路径,比如:
```
./get_frames video.mp4
```
程序将输出视频文件的基本信息,包括总帧数。
阅读全文
相关推荐




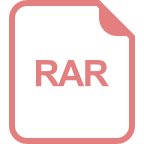

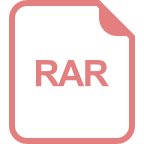











