c语言调用ffmpeg进行视频抽帧
时间: 2023-12-02 18:30:42 浏览: 69
要在C语言中使用FFmpeg进行视频抽帧,需要使用FFmpeg的API。以下是一个简单的示例代码,用于从视频中提取一帧并将其保存为图像文件。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavutil/imgutils.h>
#include <libavutil/samplefmt.h>
#include <libavutil/timestamp.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
int main(int argc, char *argv[]) {
AVFormatContext *format_ctx = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVFrame *frame = NULL;
AVPacket packet;
int video_stream_index = -1;
int ret, got_frame;
// Open the video file
if (avformat_open_input(&format_ctx, argv[1], NULL, NULL) < 0) {
fprintf(stderr, "Could not open input file '%s'\n", argv[1]);
exit(EXIT_FAILURE);
}
// Retrieve stream information
if (avformat_find_stream_info(format_ctx, NULL) < 0) {
fprintf(stderr, "Could not find stream information\n");
exit(EXIT_FAILURE);
}
// Find the first video stream
for (int i = 0; i < format_ctx->nb_streams; i++) {
if (format_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
fprintf(stderr, "Could not find video stream\n");
exit(EXIT_FAILURE);
}
// Get the codec parameters for the video stream
AVCodecParameters *codec_params = format_ctx->streams[video_stream_index]->codecpar;
// Find the decoder for the video stream
codec = avcodec_find_decoder(codec_params->codec_id);
if (!codec) {
fprintf(stderr, "Codec not found\n");
exit(EXIT_FAILURE);
}
// Allocate a codec context for the decoder
codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
fprintf(stderr, "Could not allocate codec context\n");
exit(EXIT_FAILURE);
}
// Copy codec parameters to codec context
if (avcodec_parameters_to_context(codec_ctx, codec_params) < 0) {
fprintf(stderr, "Could not copy codec parameters to codec context\n");
exit(EXIT_FAILURE);
}
// Open the codec
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
fprintf(stderr, "Could not open codec\n");
exit(EXIT_FAILURE);
}
// Allocate a frame for decoding
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Could not allocate frame\n");
exit(EXIT_FAILURE);
}
// Read frames from the file
while (av_read_frame(format_ctx, &packet) >= 0) {
// Check if the packet belongs to the video stream
if (packet.stream_index == video_stream_index) {
// Decode the video frame
ret = avcodec_decode_video2(codec_ctx, frame, &got_frame, &packet);
if (ret < 0) {
fprintf(stderr, "Error decoding video frame\n");
exit(EXIT_FAILURE);
}
// If a frame was decoded, convert it to RGB and save as a PNG image
if (got_frame) {
AVFrame *rgb_frame = av_frame_alloc();
int num_bytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, codec_ctx->width, codec_ctx->height, 1);
uint8_t *buffer = av_malloc(num_bytes * sizeof(uint8_t));
av_image_fill_arrays(rgb_frame->data, rgb_frame->linesize, buffer, AV_PIX_FMT_RGB24, codec_ctx->width, codec_ctx->height, 1);
struct SwsContext *sws_ctx = sws_getContext(
codec_ctx->width, codec_ctx->height, codec_ctx->pix_fmt,
codec_ctx->width, codec_ctx->height, AV_PIX_FMT_RGB24,
SWS_BILINEAR, NULL, NULL, NULL);
sws_scale(sws_ctx, frame->data, frame->linesize, 0, codec_ctx->height, rgb_frame->data, rgb_frame->linesize);
sws_freeContext(sws_ctx);
char filename[32];
snprintf(filename, sizeof(filename), "frame-%d.png", frame->display_picture_number);
av_write_image_header(rgb_frame, filename);
av_write_image_frame(format_ctx, rgb_frame);
av_freep(&rgb_frame->data[0]);
av_frame_free(&rgb_frame);
}
}
av_packet_unref(&packet);
}
// Clean up
avcodec_free_context(&codec_ctx);
av_frame_free(&frame);
avformat_close_input(&format_ctx);
avformat_free_context(format_ctx);
return 0;
}
```
在这个示例中,首先打开视频文件,并查找第一个视频流。然后,获取该流的编解码器参数,并使用它们创建一个编解码器上下文。接下来,打开编解码器,并为解码分配一个帧。然后,循环读取文件中的数据包,并对视频流进行解码。如果成功解码了一帧,它将被转换为RGB格式,并保存为PNG图像文件。最后,清理所有使用的资源。
请注意,此示例仅提供一个基本框架,您可能需要根据您的具体需求进行修改和调整。
相关推荐
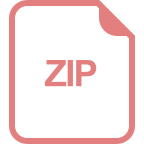
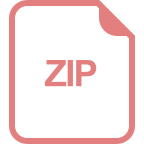














