用C语言调用ffmpeg的api读取文件的像素数据
时间: 2023-05-29 21:03:51 浏览: 196
以下是使用C语言调用FFmpeg的API读取文件的像素数据的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <libavutil/imgutils.h>
#include <libavutil/parseutils.h>
#include <libavformat/avformat.h>
int main(int argc, char **argv)
{
AVFormatContext *fmt_ctx = NULL;
int video_stream_index = -1;
AVCodecParameters *codec_params = NULL;
AVCodec *decoder = NULL;
AVCodecContext *decoder_ctx = NULL;
AVFrame *frame = NULL;
int ret, i;
if (argc < 2) {
fprintf(stderr, "Usage: %s <input_file>\n", argv[0]);
return 1;
}
// Open input file
ret = avformat_open_input(&fmt_ctx, argv[1], NULL, NULL);
if (ret < 0) {
fprintf(stderr, "Failed to open input file: %s\n", av_err2str(ret));
goto end;
}
// Read stream information
ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0) {
fprintf(stderr, "Failed to read stream information: %s\n", av_err2str(ret));
goto end;
}
// Find video stream
for (i = 0; i < fmt_ctx->nb_streams; i++) {
if (fmt_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
fprintf(stderr, "No video stream found in input file\n");
goto end;
}
// Get codec parameters
codec_params = fmt_ctx->streams[video_stream_index]->codecpar;
// Find decoder
decoder = avcodec_find_decoder(codec_params->codec_id);
if (!decoder) {
fprintf(stderr, "Failed to find decoder for codec ID %d\n", codec_params->codec_id);
goto end;
}
// Allocate decoder context
decoder_ctx = avcodec_alloc_context3(decoder);
if (!decoder_ctx) {
fprintf(stderr, "Failed to allocate decoder context\n");
goto end;
}
// Copy codec parameters to decoder context
ret = avcodec_parameters_to_context(decoder_ctx, codec_params);
if (ret < 0) {
fprintf(stderr, "Failed to copy codec parameters to decoder context: %s\n", av_err2str(ret));
goto end;
}
// Open decoder
ret = avcodec_open2(decoder_ctx, decoder, NULL);
if (ret < 0) {
fprintf(stderr, "Failed to open decoder: %s\n", av_err2str(ret));
goto end;
}
// Allocate frame
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Failed to allocate frame\n");
goto end;
}
// Read frames from input file
while (1) {
AVPacket pkt = {0};
av_init_packet(&pkt);
ret = av_read_frame(fmt_ctx, &pkt);
if (ret == AVERROR_EOF)
break;
if (ret < 0) {
fprintf(stderr, "Failed to read frame: %s\n", av_err2str(ret));
goto end;
}
// Decode packet
ret = avcodec_send_packet(decoder_ctx, &pkt);
if (ret < 0) {
fprintf(stderr, "Failed to send packet to decoder: %s\n", av_err2str(ret));
goto end;
}
while (ret >= 0) {
ret = avcodec_receive_frame(decoder_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
if (ret < 0) {
fprintf(stderr, "Failed to receive frame from decoder: %s\n", av_err2str(ret));
goto end;
}
// Process frame
printf("Frame %d (type=%c, size=%d bytes) pts %d key_frame %d\n",
decoder_ctx->frame_number, av_get_picture_type_char(frame->pict_type),
frame->pkt_size, frame->pts, frame->key_frame);
// Process pixel data
uint8_t *data[AV_NUM_DATA_POINTERS] = {0};
int linesize[AV_NUM_DATA_POINTERS] = {0};
int width, height;
data[0] = frame->data[0];
linesize[0] = frame->linesize[0];
width = frame->width;
height = frame->height;
// Process Y component
for (i = 0; i < height; i++) {
fwrite(data[0] + i * linesize[0], 1, width, stdout);
}
// Process U component
if (codec_params->format == AV_PIX_FMT_YUV420P) {
data[1] = frame->data[1];
linesize[1] = frame->linesize[1];
for (i = 0; i < height/2; i++) {
fwrite(data[1] + i * linesize[1], 1, width/2, stdout);
}
}
// Process V component
if (codec_params->format == AV_PIX_FMT_YUV420P) {
data[2] = frame->data[2];
linesize[2] = frame->linesize[2];
for (i = 0; i < height/2; i++) {
fwrite(data[2] + i * linesize[2], 1, width/2, stdout);
}
}
printf("\n");
av_frame_unref(frame);
}
av_packet_unref(&pkt);
}
end:
if (frame)
av_frame_free(&frame);
if (decoder_ctx)
avcodec_free_context(&decoder_ctx);
if (fmt_ctx)
avformat_close_input(&fmt_ctx);
return ret;
}
```
此代码可读取输入文件中的视频帧,然后处理像素数据并将其写入stdout。在处理像素数据时,该代码将Y、U和V分量分别写入stdout。在处理像素数据时,还应注意缩放、旋转和其他处理,以使像素数据适合应用程序的需要。
阅读全文
相关推荐
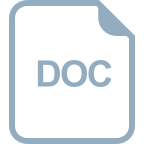
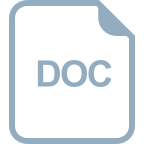
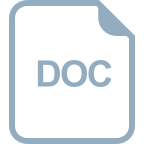





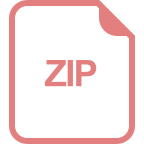
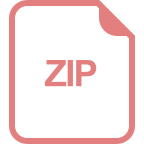




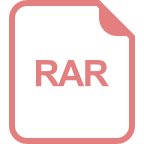
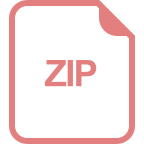
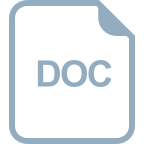
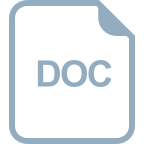
