accessor and mutator c++在对象中如何使用
时间: 2024-05-01 09:18:07 浏览: 13
Accessor和Mutator在面向对象编程中通常是用于访问和修改对象属性的方法。在C语言中,我们可以使用结构体来模拟对象,然后在结构体中定义成员变量和成员函数来实现Accessor和Mutator的功能。具体地说,我们可以在结构体中声明public和private的成员变量,然后通过public的成员函数来访问和修改private成员变量,这样就保证了数据的封装性和安全性。例如:
```
//定义一个结构体来模拟对象
typedef struct {
int age; //private成员变量
} Person;
//定义public的成员函数来访问和修改private成员变量
int get_age(Person *p) {
return p->age;
}
void set_age(Person *p, int age) {
p->age = age;
}
```
这样,我们就可以在C语言中实现类似于Accessor和Mutator的功能了。
相关问题
Define a Pet class that stores the pet’s name, age, and weight. Add appropriate constructors, accessor, and mutator functions. Also define a function named getLifespan that returns a string with the value “unknown lifespan.”
Sure! Here's an example implementation of the `Pet` class in Python:
```python
class Pet:
def __init__(self, name, age, weight):
self.name = name
self.age = age
self.weight = weight
# Accessor functions
def getName(self):
return self.name
def getAge(self):
return self.age
def getWeight(self):
return self.weight
# Mutator functions
def setName(self, name):
self.name = name
def setAge(self, age):
self.age = age
def setWeight(self, weight):
self.weight = weight
# Additional function
def getLifespan(self):
return "unknown lifespan"
```
In this implementation, the `Pet` class has a constructor that takes in the pet's name, age, and weight as parameters and initializes the corresponding instance variables. It also has accessor and mutator functions for each instance variable.
The `getLifespan` function is defined to return the string "unknown lifespan" as per the requirements. Note that this function does not take any parameters and is not an accessor or mutator function.
DEVC++ Define a Pet class that stores the pet’s name, age, and weight. Add appropriate constructors, accessor, and mutator functions. Also define a function named getLifespan that returns a string with the value “unknown lifespan.” Next, define a Dog class that is derived from Pet. The Dog class should have a private member variable named breed that stores the breed of the dog. Add mutator and accessor functions for the breed variable and appropriate constructors. Redefine the getLifespan function to return "Approximately 7 years" if the dog’s weight is over 100 pounds and "Approximately 13 years" if the dog’s weight is under 100 pounds. Next, define a Rock class that is derived from Pet. Redefine the getLifespan function to return “Thousands of years”. Finally, write a test program that creates instances of pet rocks and pet dogs that exercise the inherited and redefined functions.
Here is a possible implementation of the Pet, Dog, and Rock classes in C++:
```cpp
#include <iostream>
#include <string>
class Pet {
public:
Pet(const std::string& name, int age, double weight)
: name(name), age(age), weight(weight) {}
virtual ~Pet() {}
const std::string& getName() const { return name; }
int getAge() const { return age; }
double getWeight() const { return weight; }
void setName(const std::string& newName) { name = newName; }
void setAge(int newAge) { age = newAge; }
void setWeight(double newWeight) { weight = newWeight; }
virtual std::string getLifespan() const { return "unknown lifespan"; }
private:
std::string name;
int age;
double weight;
};
class Dog : public Pet {
public:
Dog(const std::string& name, int age, double weight, const std::string& breed)
: Pet(name, age, weight), breed(breed) {}
const std::string& getBreed() const { return breed; }
void setBreed(const std::string& newBreed) { breed = newBreed; }
virtual std::string getLifespan() const override {
if (getWeight() > 100) {
return "Approximately 7 years";
} else {
return "Approximately 13 years";
}
}
private:
std::string breed;
};
class Rock : public Pet {
public:
Rock(const std::string& name, int age, double weight)
: Pet(name, age, weight) {}
virtual std::string getLifespan() const override {
return "Thousands of years";
}
};
int main() {
Dog dog("Fido", 5, 80, "Golden Retriever");
std::cout << dog.getName() << " is a " << dog.getBreed()
<< " and lives for " << dog.getLifespan() << std::endl;
Rock rock("Pebbles", 1000, 0.1);
std::cout << rock.getName() << " is a rock and lives for "
<< rock.getLifespan() << std::endl;
return 0;
}
```
In this implementation, the Pet class stores the basic information about a pet, such as its name, age, and weight, and provides accessor and mutator functions for these variables. The getLifespan function is virtual and returns a default value of "unknown lifespan". The Dog class adds a private member variable for the breed of the dog, as well as mutator and accessor functions for it. It also redefines the getLifespan function to return a different value depending on the weight of the dog. The Rock class is a special case of a pet that has a very long lifespan, so it redefines the getLifespan function accordingly.
The main function creates instances of a dog and a rock, and calls their member functions to exercise their behavior. The output of the program should be:
```
Fido is a Golden Retriever and lives for Approximately 13 years
Pebbles is a rock and lives for Thousands of years
```
相关推荐
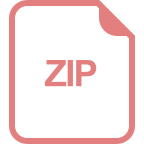









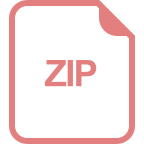
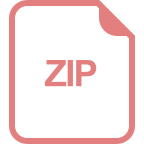
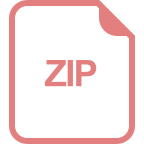
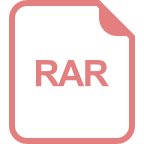