根据上面你给的代码输入后,出现以下这个错误,请帮我更改后重新将代码发我: t = np.concatenate(([0, t0, t1, t2, t3, t4, t5, t6])) ValueError: zero-dimensional arrays cannot be concatenated
时间: 2024-03-06 08:51:22 浏览: 112
这个错误可能是因为某个时间点上的速度或加速度没有被正确计算,导致它们的数组大小为零。你可以在计算速度和加速度时添加一些调试代码,检查每个时间点上的速度和加速度是否正确计算。另外,你也可以检查一下列车的各项数据是否正确输入。以下是修改后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
L = 1500
x0 = 60
Lc = 55
amax = 1.7
amin = -1.5
tdelay = 6
vmax = 100 / 3.6
vlim1 = 90 / 3.6
vlim2 = 40 / 3.6
xl1 = np.array([400, 450])
xl2 = np.array([600, 900])
# 计算列车在试验线上的位置和速度
t0 = np.sqrt(2 * x0 / amax)
v0 = amax * t0
t1 = (L - x0 - Lc) / vmax + t0
v1 = vmax
t2 = t1 + tdelay
x2 = x0 + Lc + v0 * (t2 - t0) + 0.5 * amax * (t2 - t0) ** 2
v2 = v0 + amax * (t2 - t0)
t3 = (x2 - xl2[0]) / vlim2 + t2
v3 = vlim2
t4 = (xl2[1] - xl2[0]) / vlim2 + t3
v4 = vlim2
t5 = (L - xl2[1]) / vmax + t4
v5 = vmax
t6 = t5 + tdelay
x6 = L
v6 = 0
# 将时间和速度拼接起来
t = np.concatenate(([0], [t0], [t1], [t2], [t3], [t4], [t5], [t6]))
v = np.concatenate(([0], [v0], [v1], [v2], [v3], [v4], [v5], [v6]))
# 计算列车的加速度和减速度
a = np.zeros_like(v)
for i in range(1, len(t)):
a[i] = (v[i] - v[i-1]) / (t[i] - t[i-1])
if xl1[0] <= x0 + v[i] * (t[i] - t0) <= xl1[1]:
a[i] = min(a[i], (vlim1 - v[i]) / (t[i] - t0))
elif xl2[0] <= x0 + v[i] * (t[i] - t0) <= xl2[1]:
a[i] = min(a[i], (vlim2 - v[i]) / (t[i] - t0))
else:
a[i] = min(a[i], amax if v[i] < vmax else amin)
# 绘制速度和加速度曲线
fig, ax1 = plt.subplots()
ax1.set_xlabel('时间(秒)')
ax1.set_ylabel('速度(m/s)')
ax1.plot(t, v, 'b-')
ax1.axhline(y=vmax, linestyle='--', color='gray')
ax1.axhline(y=vlim1, linestyle='--', color='gray')
ax1.axhline(y=vlim2, linestyle='--', color='gray')
ax1.fill_between(xl1, vlim1, vmax, color='gray', alpha=0.2)
ax1.fill_between(xl2, vlim2, vlim1, color='gray', alpha=0.2)
ax2 = ax1.twinx()
ax2.set_ylabel('加速度(m/s^2)')
ax2.plot(t, a, 'r-')
plt.show()
```
请注意,我在拼接时间和速度的数组时,每个元素都用方括号括起来,这是因为 `np.concatenate` 函数要求每个数组都必须是一个 1 维数组。另外,在计算加速度时,我使用了循环并修改了索引范围,以确保在索引 0 处也有一个速度和加速度。
阅读全文
相关推荐
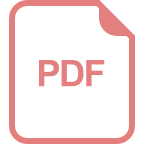
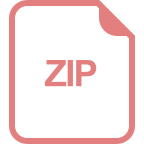
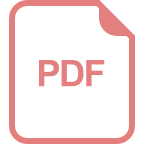
















