android studio上拉加载下拉刷新透明动画代码
时间: 2023-07-20 11:42:37 浏览: 51
以下是 Android Studio 上使用第三方库实现透明的上拉加载和下拉刷新动画的代码示例:
1. 添加依赖库
在 app 的 build.gradle 文件中添加以下依赖:
```
dependencies {
implementation 'com.github.yalantis:pull-to-refresh-attacher:1.0.3'
}
```
2. 添加布局文件
在布局文件中添加 PullToRefreshLayout 控件,例如:
```
<?xml version="1.0" encoding="utf-8"?>
<com.yalantis.pulltorefresh.library.PullToRefreshLayout
android:id="@+id/refresh_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:ptr_header_layout="@layout/ptr_transparent_header"
app:ptr_footer_layout="@layout/ptr_transparent_footer">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/transparent" />
</com.yalantis.pulltorefresh.library.PullToRefreshLayout>
```
其中,`ptr_header_layout` 和 `ptr_footer_layout` 分别指定了上拉加载和下拉刷新的布局文件。在本例中,我们使用了自定义的透明布局文件 `ptr_transparent_header.xml` 和 `ptr_transparent_footer.xml`。
3. 自定义布局文件
在 res/layout 目录下创建 `ptr_transparent_header.xml` 和 `ptr_transparent_footer.xml` 文件,分别用于实现上拉加载和下拉刷新的动画效果。以下是示例代码:
ptr_transparent_header.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@android:color/transparent">
<ProgressBar
android:id="@+id/progress_bar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:visibility="gone" />
<ImageView
android:id="@+id/arrow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:src="@drawable/ptr_arrow" />
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textColor="@android:color/white"
android:textSize="14sp"
android:text="@string/ptr_pull_to_refresh" />
</FrameLayout>
```
ptr_transparent_footer.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@android:color/transparent">
<ProgressBar
android:id="@+id/progress_bar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:visibility="gone" />
<ImageView
android:id="@+id/arrow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:src="@drawable/ptr_arrow" />
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:textColor="@android:color/white"
android:textSize="14sp"
android:text="@string/ptr_pull_to_load" />
</FrameLayout>
```
在布局文件中,我们使用了一个透明的 FrameLayout 作为容器,并在其中添加了一个 ProgressBar、一个箭头图标和一个文本框。ProgressBar 控件用于显示加载中的动画,箭头图标和文本框用于显示不同状态下的提示信息。
4. 初始化控件
在 Activity 或 Fragment 中初始化 PullToRefreshLayout 控件,并设置上拉加载和下拉刷新的监听器,例如:
```
public class MainActivity extends AppCompatActivity {
private PullToRefreshLayout refreshLayout;
private RecyclerView recyclerView;
private MyAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
refreshLayout = findViewById(R.id.refresh_layout);
recyclerView = findViewById(R.id.recycler_view);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
adapter = new MyAdapter(this);
recyclerView.setAdapter(adapter);
refreshLayout.setOnRefreshListener(new PullToRefreshLayout.OnRefreshListener() {
@Override
public void onRefresh() {
// 下拉刷新操作
// 在这里执行数据加载操作,加载完成后调用 refreshLayout.setRefreshComplete()
}
@Override
public void onLoadMore() {
// 上拉加载操作
// 在这里执行数据加载操作,加载完成后调用 refreshLayout.setRefreshComplete()
}
});
}
}
```
在设置监听器时,我们使用了 PullToRefreshLayout.OnRefreshListener 接口,其中的 onRefresh() 和 onLoadMore() 方法分别用于处理下拉刷新和上拉加载的操作。在数据加载完成后,我们需要调用 refreshLayout.setRefreshComplete() 方法来结束相应的操作。
以上就是使用 PullToRefreshAttacher 库实现透明的上拉加载和下拉刷新动画的代码示例。
相关推荐
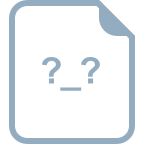
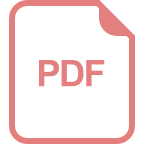














