已知类ArrayList实现了List接口,欲创建ArrayList类的一个对象
时间: 2023-08-20 19:20:55 浏览: 39
要创建ArrayList类的一个对象,可以使用如下代码:
```
List<String> arrayList = new ArrayList<String>();
```
这里使用了多态的概念,将ArrayList类的对象赋值给List接口的引用变量。这样做的好处是,如果以后需要更换具体实现类,只需要修改一处代码就可以了,而不需要修改多处代码。此外,使用泛型可以指定ArrayList中存储的元素类型,这样可以在编译时就检查类型的正确性,避免在运行时出现类型转换异常等问题。
相关问题
已知一个Bag类
好的,您已知一个Bag类,我来简单介绍一下Java中的Bag类。
在Java中,Bag类通常用来表示一个集合,它可以存储任意类型的元素,并且允许元素重复。Bag类中提供了一系列方法来操作集合中的元素,例如添加、删除、查找等。
下面是一个简单的Bag类的示例代码:
```
public class Bag<T> {
private List<T> items;
public Bag() {
items = new ArrayList<>();
}
public void add(T item) {
items.add(item);
}
public boolean remove(T item) {
return items.remove(item);
}
public boolean contains(T item) {
return items.contains(item);
}
public int size() {
return items.size();
}
public List<T> getItems() {
return items;
}
// 其他方法...
}
```
在这个Bag类中,我们使用了泛型类型参数T来表示集合中元素的类型。我们使用一个List来存储集合中的元素,通过add、remove、contains等方法来操作集合中的元素。其中,getItems方法返回整个集合的列表,size方法返回集合的大小。
需要注意的是,Bag类中允许元素重复,因此在查找元素时可能会返回多个结果。如果需要保证元素不重复,可以使用Set类来代替Bag类。
总之,Bag类是Java中表示集合的一种方式,它可以存储任意类型的元素,并且允许元素重复。在使用Bag类时,需要根据具体的需求来选择合适的集合类型。
已知ArrayList是非线程安全的,完成创建一个当前集合的线程安全版本集合的代码
可以使用以下代码创建一个线程安全版本的ArrayList:
```
List<Object> synchronizedList = Collections.synchronizedList(new ArrayList<Object>());
```
这里使用了Collections类的synchronizedList方法来创建一个同步的ArrayList,这样就可以保证在多线程环境下对该列表的访问是线程安全的。
相关推荐
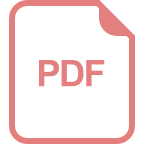
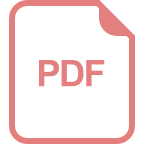
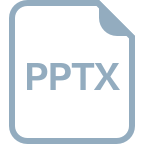












