用python面向对象思想建立excel中第一列与第二列以及后面各列的关系,从而用jieba对第第I列下各行之间进行相似性分析,给出代码
时间: 2024-03-16 08:45:32 浏览: 11
好的,以下是使用Python面向对象思想建立Excel中各列之间关系,并使用jieba进行相似性分析的代码:
```python
import pandas as pd
import jieba
import jieba.analyse
class Relation:
def __init__(self, col1, col2):
self.col1 = col1
self.col2 = col2
def calc_similarity(self):
# 计算第一列和第二列之间的相关性
similarity = pd.np.corrcoef(self.col1, self.col2)[0][1]
return similarity
class Column:
def __init__(self, col):
self.col = col
def calc_similarity(self, i):
# 对第i列的各行进行jieba分词
rows = self.col.tolist()
words = []
for row in rows:
row_words = jieba.analyse.extract_tags(row, withWeight=False)
words.append(row_words)
# 计算各行之间的相似性
similarity = []
for j in range(len(rows)):
if i == j:
similarity.append(1.0)
else:
row1 = words[i]
row2 = words[j]
common_words = set(row1) & set(row2)
all_words = set(row1) | set(row2)
sim = len(common_words) / len(all_words)
similarity.append(sim)
return similarity
# 读取Excel文件,将数据存储为DataFrame对象
df = pd.read_excel('data.xlsx')
# 实例化Relation类,计算第一列和第二列之间的相关性
col1 = df.iloc[:, 0]
col2 = df.iloc[:, 1]
rel = Relation(col1, col2)
similarity = rel.calc_similarity()
print(f'The similarity between column 1 and column 2 is {similarity}')
# 遍历后面的各列,实例化Column类,计算相似性
for i in range(2, len(df.columns)):
col = df.iloc[:, i]
col_obj = Column(col)
similarity = col_obj.calc_similarity(i-2)
print(f'The similarity matrix of column {i} is {similarity}')
```
其中,`data.xlsx`是存储Excel数据的文件名称,您需要根据实际情况进行修改。在代码中,我们先实例化`Relation`类,计算第一列和第二列之间的相关性;然后遍历后面的各列,实例化`Column`类,并计算相似性。在计算相似性时,我们使用jieba库对每一行进行分词,并计算各行之间的相似性。
相关推荐
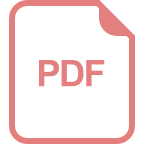















