基于html,css,php,写一个登录页面和注册页面,且输入账号和密码正确后,登陆页面可以跳转到index.html
时间: 2023-09-15 10:19:36 浏览: 64
好的,以下是一个简单的登录页面和注册页面的示例。假设你已经有一个名为 "index.html" 的主页文件。
登录页面代码:
```
<!DOCTYPE html>
<html>
<head>
<title>Login Page</title>
<style>
form {
margin: 50px auto;
width: 300px;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[type="text"], input[type="password"] {
width: 100%;
margin-bottom: 10px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
input[type="submit"] {
width: 100%;
padding: 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<form method="post" action="login.php">
<h2>Login</h2>
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<input type="submit" value="Login">
</form>
</body>
</html>
```
注册页面代码:
```
<!DOCTYPE html>
<html>
<head>
<title>Registration Page</title>
<style>
form {
margin: 50px auto;
width: 300px;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[type="text"], input[type="password"] {
width: 100%;
margin-bottom: 10px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
input[type="submit"] {
width: 100%;
padding: 10px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<form method="post" action="register.php">
<h2>Register</h2>
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<input type="submit" value="Register">
</form>
</body>
</html>
```
在这两个页面中,我们使用了 HTML 和 CSS 来创建表单。在登录页面中,我们使用了 PHP 来处理用户提交的表单数据,并验证用户输入的用户名和密码是否正确,如果正确,则将用户重定向到 "index.html" 页面。
以下是一个简单的 "login.php" 文件,用于处理登录表单:
```
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
// 获取表单数据
$username = $_POST['username'];
$password = $_POST['password'];
// 假设合法用户为 "admin",密码为 "123456"
if ($username === 'admin' && $password === '123456') {
// 跳转到主页
header('Location: index.html');
exit;
} else {
echo 'Invalid username or password.';
}
}
?>
```
同样地,我们也可以创建一个 "register.php" 文件,用于处理注册表单。在这个文件中,我们可以将新用户的用户名和密码保存到数据库或文件中,用于以后的登录验证。
当用户提交表单时,我们可以使用 PHP 来处理表单数据,并在需要时将用户重定向到其他页面。在这个示例中,我们使用了简单的条件语句来验证用户输入的用户名和密码是否正确,但在实际应用中,我们可能需要使用更复杂的逻辑来验证用户输入。
相关推荐
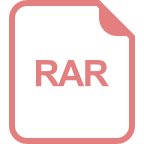
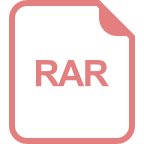
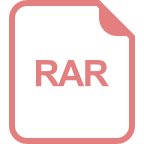
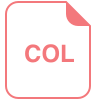
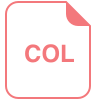
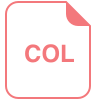
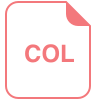
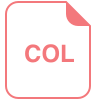









