用python生成一个GUi,具备以下功能: 1.界面内包含数据文件读取、表格中显示读取的数据以及绘图区域; 2.选择数据文件后,在表格中显示对应的数据,支持表格中数据的编辑和报存。 3.通过点击按钮可新增绘图子页面。 3.绘图区域分两部分,左侧为绘制的图片名称,右侧为绘制的图片。可通过点击某按钮增加新的绘图。
时间: 2023-05-30 14:02:03 浏览: 145
以下是一个可能的实现,使用了tkinter和matplotlib库:
```python
import tkinter as tk
import pandas as pd
from matplotlib.figure import Figure
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg
class App:
def __init__(self, master):
self.master = master
master.title("Data Viewer")
# widgets for data file selection and display
self.file_label = tk.Label(master, text="Select data file:")
self.file_label.pack()
self.file_entry = tk.Entry(master)
self.file_entry.pack()
self.load_button = tk.Button(master, text="Load", command=self.load_data)
self.load_button.pack()
self.save_button = tk.Button(master, text="Save", command=self.save_data, state=tk.DISABLED)
self.save_button.pack()
self.table = tk.Frame(master)
self.table.pack()
# widgets for plotting
self.plot_label = tk.Label(master, text="Plots:")
self.plot_label.pack()
self.plot_buttons = tk.Frame(master)
self.plot_buttons.pack()
self.plot_area = tk.Frame(master)
self.plot_area.pack()
# initialize data and plots
self.data = None
self.plots = []
def load_data(self):
# read data from file and display in table
filename = self.file_entry.get()
self.data = pd.read_csv(filename)
self.save_button.config(state=tk.NORMAL)
for widget in self.table.winfo_children():
widget.destroy()
for i, col in enumerate(self.data.columns):
tk.Label(self.table, text=col).grid(row=0, column=i)
for i, row in self.data.iterrows():
for j, val in enumerate(row):
entry = tk.Entry(self.table)
entry.grid(row=i+1, column=j)
entry.insert(0, str(val))
def save_data(self):
# save edited data to file
for i, row in self.data.iterrows():
for j, col in enumerate(self.data.columns):
val = self.table.grid_slaves(row=i+1, column=j)[0].get()
self.data.at[i, col] = val
self.data.to_csv(self.file_entry.get(), index=False)
def add_plot(self):
# create new plot and add to list of plots
fig = Figure(figsize=(5, 4), dpi=100)
ax = fig.add_subplot(111)
canvas = FigureCanvasTkAgg(fig, master=self.plot_area)
canvas.get_tk_widget().pack(side=tk.RIGHT)
self.plots.append((canvas, ax))
# create button to switch to new plot
button = tk.Button(self.plot_buttons, text=f"Plot {len(self.plots)}", command=lambda: self.switch_plot(len(self.plots)))
button.pack(side=tk.LEFT)
def switch_plot(self, index):
# switch to selected plot
for i, (canvas, ax) in enumerate(self.plots):
if i == index-1:
canvas.get_tk_widget().pack(side=tk.RIGHT)
ax.set_title(f"Plot {index}")
else:
canvas.get_tk_widget().pack_forget()
# create and run application
root = tk.Tk()
app = App(root)
app.add_plot() # add initial plot
root.mainloop()
```
阅读全文
相关推荐
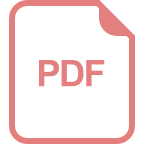
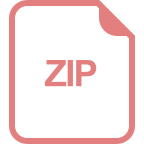
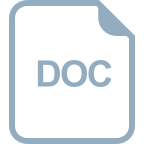
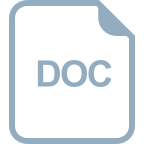
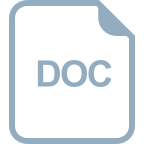
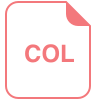
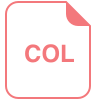
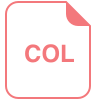







