pytorch代码实现两个损失函数加权和
时间: 2023-10-07 07:08:47 浏览: 48
可以使用如下的代码实现两个损失函数加权和:
```python
import torch
# 定义两个损失函数
loss1 = torch.nn.CrossEntropyLoss()
loss2 = torch.nn.MSELoss()
# 定义损失函数的权重
weight1 = 0.7
weight2 = 0.3
# 计算加权损失函数
def weighted_loss(loss1, loss2, weight1, weight2, output, target):
loss = weight1 * loss1(output, target) + weight2 * loss2(output, target)
return loss
# 使用示例
output = torch.randn(10, 2)
target = torch.randint(2, size=(10,))
loss = weighted_loss(loss1, loss2, weight1, weight2, output, target)
print(loss.item())
```
该代码实现了使用两个损失函数的加权和来计算总体损失函数,其中`weight1`和`weight2`为两个损失函数的权重。可以根据需求修改权重的值来平衡两个损失函数对总体损失的影响。
相关问题
wbce损失函数pytorch实现
以下是一个简单的 PyTorch 实现 WBCE 损失函数的示例代码:
```python
import torch
def weighted_binary_cross_entropy(output, target, weights=None):
if weights is not None:
assert len(weights) == 2
loss = F.binary_cross_entropy(output, target, reduction='none')
if weights is not None:
assert (weights >= 0).all()
assert weights[0] + weights[1] > 0
loss = weights[1] * target * loss + weights[0] * (1 - target) * loss
else:
loss = torch.mean(loss)
return loss
```
其中,`output` 是模型的输出,`target` 是目标值。`weights` 是权重,是一个长度为 2 的列表,包含两个元素,分别是负样本和正样本的权重。
该函数首先使用 PyTorch 自带的二分类交叉熵函数 `F.binary_cross_entropy` 计算二分类交叉熵损失,然后根据权重对损失进行加权。如果没有传入权重,则对所有样本的损失取平均值。最后返回计算得到的损失。
交叉熵损失函数在pytorch中参数说明
在PyTorch中,交叉熵损失函数`nn.CrossEntropyLoss()`的参数说明如下:
1. `weight`: 对每个类别的损失进行加权,默认为None。
2. `size_average`: 如果为True,则损失会被平均到每个样本上,默认为True。
3. `ignore_index`: 指定忽略的目标类别的索引,默认为-100。
4. `reduce`: 如果为False,则返回每个样本的损失,默认为True。
5. `reduction`: 指定如何计算损失的值。可选择的值有`'none'`、`'mean'`、`'sum'`,默认为`'mean'`。
需要注意的是,`nn.CrossEntropyLoss()`结合了`nn.LogSoftmax()`和`nn.NLLLoss()`两个函数,因此不需要在模型的输出端添加`nn.LogSoftmax()`层。此外,输入的形状应为(batch_size, num_classes)。
以下是一个示例代码,展示如何使用交叉熵损失函数:
```python
import torch
import torch.nn as nn
# 创建模型的输出和目标张量
output = torch.tensor([[0.5, 0.2, 0.3], [0.1, 0.8, 0.1]])
target = torch.tensor([0, 1]) # 目标类别的索引
# 实例化交叉熵损失函数
criterion = nn.CrossEntropyLoss()
# 计算损失
loss = criterion(output, target)
print(loss) # 输出损失的值
```
这段代码中,`output`是模型的输出,`target`是目标类别的索引。使用`nn.CrossEntropyLoss()`计算输出和目标之间的交叉熵损失,并将结果存储在`loss`中。最后打印出损失的值。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
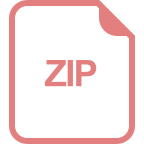
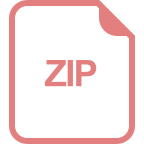
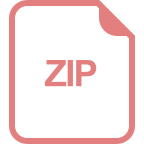
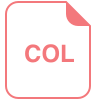
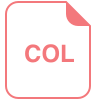
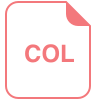
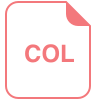
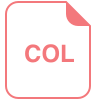







