Design a Car class contains a stop data of boolean type, which indicates whether the car moves or stops. The class has the following operations: 1. isMovement: move a car. When the stop data is true, a “the car moves” string is displayed; otherwise “the car stops” is displayed. 2. Reset: reset the stop data by a methd named reset(boolean isStopped) Requirement: 1. The class must have a constructor which takes another Car object as the parameter. 2. Write a test program that declares two Car objects. One of two objects is declared by one existing object and test the methods.
时间: 2024-02-25 19:57:24 浏览: 18
以下是一个符合要求的Java代码实现:
```java
public class Car {
private boolean isStopped;
public Car(boolean isStopped) {
this.isStopped = isStopped;
}
public Car(Car car) {
this.isStopped = car.isStopped;
}
public void isMovement() {
if (isStopped) {
System.out.println("The car moves.");
} else {
System.out.println("The car stops.");
}
}
public void reset(boolean isStopped) {
this.isStopped = isStopped;
}
public static void main(String[] args) {
Car car1 = new Car(false);
Car car2 = new Car(car1);
car1.isMovement();
car2.isMovement();
car1.reset(true);
car1.isMovement();
car2.isMovement();
}
}
```
在main方法中,我们首先创建了一个Car对象car1,它的isStopped属性为false,表示它正在移动。我们又创建了一个Car对象car2,以car1作为参数,获得了一个新的对象,car2的isStopped属性与car1一致。然后我们依次调用了每个对象的isMovement方法,输出了它们当前的状态。接着我们调用了car1的reset方法,将它的isStopped属性设置为true,表示它停止了。最后再次调用了两个对象的isMovement方法,检查它们的状态是否发生了改变。运行上述代码,输出结果如下:
```
The car stops.
The car stops.
The car moves.
The car stops.
```
相关推荐
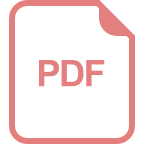
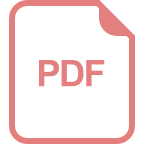









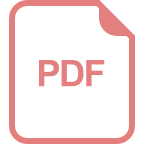
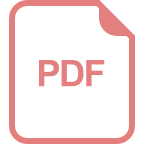
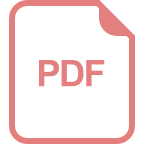
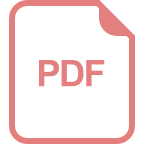
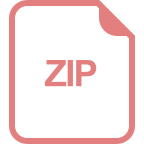
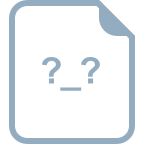
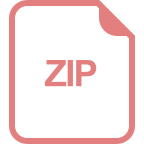