vue oss 分片上传
时间: 2023-09-30 12:07:15 浏览: 95
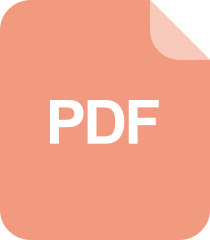
Vue2.0结合webuploader实现文件分片上传功能
Vue OSS 分片上传是指将大文件分成多个小块,分别上传到阿里云 OSS(Object Storage Service)中,最后再将这些小块合并成一个完整的文件。这样做的好处是可以避免上传大文件时出现各种问题,比如上传速度慢、网络中断等等。
下面是一个基于 Vue 和阿里云 OSS 的分片上传示例:
1. 安装阿里云 OSS SDK 和 axios:
```javascript
npm install ali-oss axios --save
```
2. 创建一个上传组件 Upload.vue:
```vue
<template>
<div>
<input type="file" ref="fileInput" @change="selectFile" />
<button @click="uploadFile">上传</button>
</div>
</template>
<script>
import OSS from 'ali-oss'
import axios from 'axios'
export default {
data() {
return {
selectedFile: null,
ossClient: null,
uploadId: null,
chunks: [],
uploadedChunks: []
}
},
methods: {
selectFile(event) {
this.selectedFile = event.target.files[0]
},
async uploadFile() {
// 初始化 OSS 客户端
this.ossClient = new OSS({
accessKeyId: 'your_access_key_id',
accessKeySecret: 'your_access_key_secret',
bucket: 'your_bucket_name',
region: 'your_region'
})
// 获取文件信息
const fileInfo = await this.ossClient.head(this.selectedFile.name)
// 计算文件分片数
const chunkSize = 1 * 1024 * 1024 // 每个分片大小为 1MB
const chunkCount = Math.ceil(fileInfo.res.headers['content-length'] / chunkSize)
// 初始化分片上传
const result = await this.ossClient.initMultipartUpload(this.selectedFile.name)
this.uploadId = result.uploadId
// 分片上传
for (let i = 0; i < chunkCount; i++) {
const start = i * chunkSize
const end = Math.min(start + chunkSize, fileInfo.res.headers['content-length'])
const chunk = this.selectedFile.slice(start, end)
this.chunks.push(chunk)
}
await Promise.all(
this.chunks.map(async (chunk, index) => {
const result = await this.ossClient.uploadPart(this.selectedFile.name, this.uploadId, index + 1, chunk)
this.uploadedChunks.push({
PartNumber: index + 1,
ETag: result.etag
})
})
)
// 完成分片上传
await this.ossClient.completeMultipartUpload(this.selectedFile.name, this.uploadId, this.uploadedChunks)
// 上传完成,发送请求到服务器
const response = await axios.post('/api/upload', {
filename: this.selectedFile.name
})
console.log(response.data)
}
}
}
</script>
```
3. 在服务器端(假设使用 Express)处理上传请求:
```javascript
const express = require('express')
const app = express()
app.post('/api/upload', (req, res) => {
const filename = req.body.filename
// 处理上传完成后的逻辑
})
app.listen(3000, () => {
console.log('Server is running at http://localhost:3000')
})
```
这样就完成了基于 Vue 和阿里云 OSS 的分片上传示例。需要注意的是,阿里云 OSS 的分片上传 API 是收费的,具体收费标准可以参考阿里云官方文档。
阅读全文
相关推荐
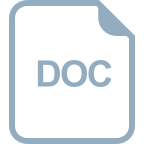
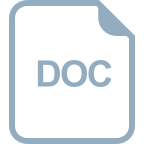






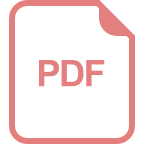
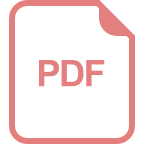
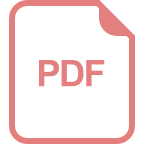
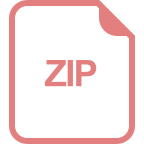
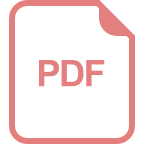
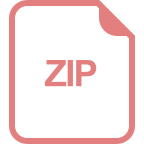
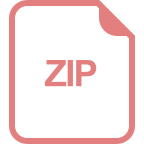
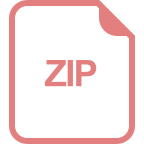
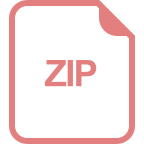