import os import shutil # 相对路径 relative_path = '.\dataset1.0\labels' # 遍历目录下的所有文件 for file_name in os.listdir(relative_path): file_path = os.path.join(relative_path, file_name) if file_name.endswith('.txt'): with open(file_path, 'r') as file: # 读取文件第一行的第一个数字作为标签类别编号 first_line = file.readline() label_number = int(first_line.strip()[0]) # 构建目标文件夹路径 destination_folder = '.\C{}'.format(label_number) # 如果目标文件夹不存在,则创建目标文件夹 if not os.path.exists(destination_folder): os.makedirs(destination_folder) # 构建目标文件路径 destination_file = os.path.join(destination_folder, file_name) # 移动文件到目标文件夹 shutil.move(file_path, destination_file)
时间: 2023-12-12 10:06:07 浏览: 150
好的,你想要将以上代码循环1000次。下面是修改后的代码:
```python
import os
import shutil
# 相对路径
relative_path = '.\dataset1.0\labels'
# 遍历目录下的所有文件
for _ in range(1000):
for file_name in os.listdir(relative_path):
file_path = os.path.join(relative_path, file_name)
if file_name.endswith('.txt'):
with open(file_path, 'r') as file:
# 读取文件第一行的第一个数字作为标签类别编号
first_line = file.readline()
label_number = int(first_line.strip()[0])
# 构建目标文件夹路径
destination_folder = '.\C{}'.format(label_number)
# 如果目标文件夹不存在,则创建目标文件夹
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
# 构建目标文件路径
destination_file = os.path.join(destination_folder, file_name)
# 移动文件到目标文件夹
shutil.move(file_path, destination_file)
```
现在以上代码会将指定路径下的文件循环1000次,每次循环都会根据读取的文件内容进行相应的操作。请确保你提供的路径和代码逻辑是正确的,并根据需要进行必要的修改。
阅读全文
相关推荐
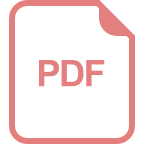











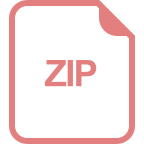