fpr,tpr, thresholds = roc_curve(y_test,clf.predict_proba(X_test)[:,1]) plt.plot(fpr,tpr,label='ROC') plt.xlabel('FPR') plt.ylabel('TPR')
时间: 2024-01-24 11:05:40 浏览: 31
这段代码是用于绘制ROC曲线的,其中:
- `roc_curve(y_test, clf.predict_proba(X_test)[:,1])`:计算二元分类的ROC曲线。`y_test`是真实标签,`clf.predict_proba(X_test)[:,1]`是模型预测的概率(这里是正例的概率)。
- `plt.plot(fpr, tpr, label='ROC')`:绘制ROC曲线,其中`fpr`是假正例率,`tpr`是真正例率。
- `plt.xlabel('FPR')`和`plt.ylabel('TPR')`:设置坐标轴标签。
ROC曲线可以用来评估一个二元分类模型的性能,曲线下面的面积越大,模型的性能越好。
相关问题
优化代码from sklearn.metrics import classification_report, roc_curve, auc # 评估分类模型性能 fpr, tpr, thresholds = roc_curve(y_test, clf.predict_proba(X_test)[:,1]) roc_auc = auc(fpr, tpr)出错ValueError: multiclass format is not supported
这个错误是因为roc_curve和auc函数不支持多分类问题的评估。你可以尝试使用OneVsRestClassifier或OneVsOneClassifier将多分类问题转化为二分类问题,然后再使用roc_curve和auc函数进行评估。
具体来说,你可以按照以下步骤进行修改:
1. 导入OneVsRestClassifier或OneVsOneClassifier:
```
from sklearn.multiclass import OneVsRestClassifier
或者
from sklearn.multiclass import OneVsOneClassifier
```
2. 将分类器包装在OneVsRestClassifier或OneVsOneClassifier中:
```
clf = OneVsRestClassifier(clf)
或者
clf = OneVsOneClassifier(clf)
```
3. 对y进行one-hot编码:
```
from sklearn.preprocessing import LabelBinarizer
lb = LabelBinarizer()
y_test = lb.fit_transform(y_test)
```
4. 使用predict_proba函数预测概率:
```
y_score = clf.predict_proba(X_test)
```
5. 调用roc_curve和auc函数进行评估:
```
fpr, tpr, thresholds = roc_curve(y_test[:, 1], y_score[:, 1])
roc_auc = auc(fpr, tpr)
```
注意,这里的y_test[:, 1]表示取出one-hot编码后的第二列,即正例的概率,y_score[:, 1]表示取出分类器预测的正例的概率。
希望能帮到你!
优化代码# 打印分类报告 print(classification_report(y_test, y_pred)) from sklearn.metrics import classification_report, roc_curve, auc # 评估分类模型性能 fpr, tpr, thresholds = roc_curve(y_test, clf.predict_proba(X_test)[:,1]) roc_auc = auc(fpr, tpr)出错ValueError: multiclass format is not supported
这个错误提示是因为 `roc_curve` 函数不支持多分类问题的评估。该函数是用于二分类问题的。如果你的模型是用于多分类问题的,则需要使用其他的评估指标。
对于多分类问题,可以使用以下指标进行评估:
- 混淆矩阵(confusion matrix)
- 准确率(accuracy)
- 精确率(precision)
- 召回率(recall)
- F1-score
- Kappa系数(Kappa coefficient)
你可以使用 `confusion_matrix` 函数来计算混淆矩阵。例如:
```python
from sklearn.metrics import confusion_matrix
y_pred = clf.predict(X_test)
cm = confusion_matrix(y_test, y_pred)
print(cm)
```
你也可以使用 `classification_report` 函数来打印准确率、精确率、召回率和 F1-score。例如:
```python
from sklearn.metrics import classification_report
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
```
Kappa系数也可以使用 `cohen_kappa_score` 函数来计算。例如:
```python
from sklearn.metrics import cohen_kappa_score
y_pred = clf.predict(X_test)
kappa = cohen_kappa_score(y_test, y_pred)
print(kappa)
```
需要注意的是,这些指标都是用于评估多分类问题的,而不是二分类问题。
相关推荐
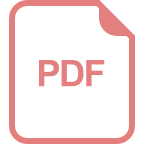
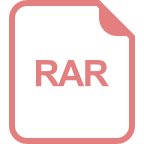
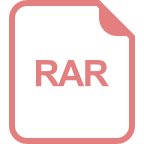












