设计一个基类(工人 Worker???)、两个派生类(司机类 Driver???、铁 匠类 Blacksmith???),在整个实验过程中???代表学号后三位。要求对这三个类 进行分析和设计,实现以下功能: 1. 所有工人都要工作 Work,但是司机开车、铁匠打铁。 2. 所有工人都要休息 Rest。 3. 休息以后,只有铁匠要洗澡 Bath。 程序输出如下: 在设计上述功能时可以考虑以下几点: 1. 要有相同的属性(成员变量)和行为(成员函数); 2. 都可以实现某一功能,但是实现的细节不一样。 3. 两个子类要有完全不同的、各自特有的行为。 请在 main 函数中通过一个基类指针数组来指向所有的派生类对象,然后通 过遍历该数组调用上述所有函数,并能显示构造函数、析构函数的调用细节。
时间: 2024-02-11 19:04:03 浏览: 32
好的,我来解答这个问题。
首先,我们需要定义一个基类 Worker,该类包含两个成员函数 Work 和 Rest,以及一个成员变量 name。
```C++
class Worker {
public:
Worker(string name) : name(name) {
cout << "Worker " << name << " is created." << endl;
}
virtual ~Worker() {
cout << "Worker " << name << " is destroyed." << endl;
}
virtual void Work() = 0;
void Rest() {
cout << "Worker " << name << " is resting." << endl;
}
protected:
string name;
};
```
该基类中定义了一个纯虚函数 Work,表示所有工人都需要工作,但是由于司机和铁匠的工作不同,因此该函数被定义成纯虚函数,具体的实现交给各自的派生类。
派生类 Driver 和 Blacksmith 分别表示司机和铁匠,它们都继承自 Worker,但是有各自独特的行为。Driver 需要开车,Blacksmith 需要打铁并且洗澡。
```C++
class Driver : public Worker {
public:
Driver(string name) : Worker(name) {
cout << "Driver " << name << " is created." << endl;
}
~Driver() {
cout << "Driver " << name << " is destroyed." << endl;
}
void Work() {
cout << "Driver " << name << " is driving." << endl;
}
};
class Blacksmith : public Worker {
public:
Blacksmith(string name) : Worker(name) {
cout << "Blacksmith " << name << " is created." << endl;
}
~Blacksmith() {
cout << "Blacksmith " << name << " is destroyed." << endl;
}
void Work() {
cout << "Blacksmith " << name << " is blacksmithing." << endl;
}
void Bath() {
cout << "Blacksmith " << name << " is bathing." << endl;
}
};
```
注意,这里的析构函数也需要分别定义,以便能够输出对象的创建和销毁过程。
现在我们可以在 main 函数中创建一个基类指针数组,其中包含了一个 Driver 对象和一个 Blacksmith 对象,并遍历该数组,分别调用 Work、Rest 和 Bath 函数。
```C++
int main() {
Worker* workers[2];
workers[0] = new Driver("001");
workers[1] = new Blacksmith("002");
for (int i = 0; i < 2; i++) {
workers[i]->Work();
workers[i]->Rest();
if (dynamic_cast<Blacksmith*>(workers[i])) {
Blacksmith* b = dynamic_cast<Blacksmith*>(workers[i]);
b->Bath();
}
}
for (int i = 0; i < 2; i++) {
delete workers[i];
}
return 0;
}
```
需要注意的是,Bath 函数只有 Blacksmith 才有,因此我们需要使用 dynamic_cast 进行类型转换,以便在调用 Bath 函数时避免出错。
最后别忘了释放内存,销毁对象。
相关推荐
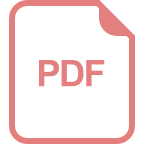
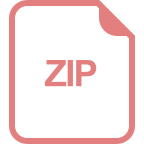
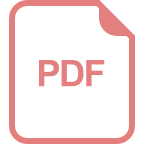















