用C++编写程序读入一段文本到vector对象,每个单词存储为vector中的一个元素。把vector对象中每个单词转化为大写字母。输出vector对象中转化后的元素,每八个单词为一行输出。
时间: 2024-05-12 22:16:51 浏览: 29
好的,让我来为您解答这个问题。下面是C语言代码实现的思路:
1. 首先,您需要打开一个文本文件,并从中读取数据。
2. 使用vector对象存储读入的每个单词,可以使用push_back()函数将读取的单词添加到vector中。
3. 遍历vector中的每个元素,使用toupper()函数将每个单词转换为大写字母。
4. 使用循环和取余运算符将vector中的元素以每八个单词一行的方式输出。
下面是实现代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <vector>
using namespace std;
int main()
{
FILE *fp;
char word[100];
fp = fopen("input.txt", "r");
if(fp == NULL)
{
printf("Error opening file\n");
exit(1);
}
vector<string> words;
while(fscanf(fp, "%s", word) == 1)
{
words.push_back(word);
}
fclose(fp);
for(int i = 0; i < words.size(); i++)
{
for(int j = 0; j < words[i].size(); j++)
{
words[i][j] = toupper(words[i][j]);
}
}
for(int i = 0; i < words.size(); i++)
{
printf("%s ", words[i].c_str());
if((i + 1) % 8 == 0)
{
printf("\n");
}
}
return 0;
}
```
希望这个答案对您有所帮助!
相关推荐
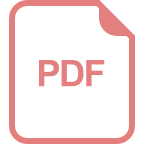
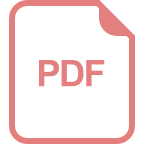














