netty 整合mybatis-plus
时间: 2023-06-14 11:04:46 浏览: 460
Net 是一款高性能的网络通信框架,MyBatis-Plus 是 MyBatis 的增强工具,在实现数据访问时提供了更加便捷的 API。将它们整合起来可以实现一个高性能的网络通信应用。
下面是整合步骤:
1. 引入 Netty 和 MyBatis-Plus 的依赖:
```xml
<!-- Netty -->
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>${netty.version}</version>
</dependency>
<!-- MyBatis-Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>${mybatis-plus.version}</version>
</dependency>
```
2. 编写 Netty 的服务端代码,实现业务逻辑处理:
```java
public class MyNettyServer {
private static final int PORT = 8888;
public void start() {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new MyNettyServerHandler());
}
});
ChannelFuture future = bootstrap.bind(PORT).sync();
future.channel().closeFuture().sync();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
```
3. 编写 Netty 的处理器 MyNettyServerHandler,实现业务逻辑:
```java
public class MyNettyServerHandler extends ChannelInboundHandlerAdapter {
@Autowired
private UserService userService;
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
ByteBuf byteBuf = (ByteBuf) msg;
String request = byteBuf.toString(CharsetUtil.UTF_8);
String response = userService.getUser(request);
ByteBuf responseBuf = Unpooled.copiedBuffer(response, CharsetUtil.UTF_8);
ctx.writeAndFlush(responseBuf);
}
}
```
4. 编写 MyBatis-Plus 的服务:
```java
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
@Override
public String getUser(String name) {
User user = getOne(new LambdaQueryWrapper<User>().eq(User::getName, name));
return user == null ? "User not found" : user.toString();
}
}
```
5. 在 Spring Boot 中整合 Netty 和 MyBatis-Plus:
```java
@SpringBootApplication
public class MyApplication implements CommandLineRunner {
@Autowired
private MyNettyServer myNettyServer;
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
myNettyServer.start();
}
}
```
至此,Netty 和 MyBatis-Plus 的整合就完成了,可以在 Netty 的处理器中调用 MyBatis-Plus 的服务实现业务逻辑。
阅读全文
相关推荐
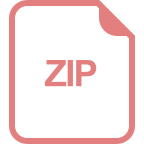
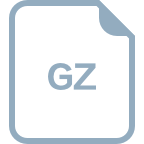
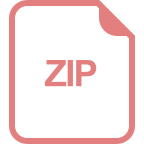















