linux根据用户名获取uid和gid的C函数
时间: 2023-12-10 15:04:50 浏览: 283
可以使用`getpwnam()`函数来获取指定用户名的用户信息,其中包括用户的uid和gid。示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <pwd.h>
int main(int argc, char *argv[]) {
struct passwd *pw;
if (argc != 2) {
fprintf(stderr, "Usage: %s username\n", argv[0]);
exit(EXIT_FAILURE);
}
pw = getpwnam(argv[1]);
if (pw == NULL) {
fprintf(stderr, "User not found: %s\n", argv[1]);
exit(EXIT_FAILURE);
}
printf("Username: %s\n", pw->pw_name);
printf("UID: %d\n", pw->pw_uid);
printf("GID: %d\n", pw->pw_gid);
return EXIT_SUCCESS;
}
```
该程序可以通过命令行参数指定要查询的用户名,然后输出该用户的用户名、UID和GID。如果用户名不存在,则输出错误信息并退出。
相关问题
实验 6仿写 Linux 下的 ls-l 命令,需要显示的文件属性包括:文件类型、权限、硬链接数、所有者用户名、 所有者所在组用户名、文件大小和最后修改时间。,要求用c语言
以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#include <pwd.h>
#include <grp.h>
#include <time.h>
int main(int argc, char *argv[]) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <directory>\n", argv[0]);
exit(EXIT_FAILURE);
}
DIR *dir;
struct dirent *entry;
struct stat sb;
char buf[512];
if ((dir = opendir(argv[1])) == NULL) {
perror("opendir");
exit(EXIT_FAILURE);
}
while ((entry = readdir(dir)) != NULL) {
sprintf(buf, "%s/%s", argv[1], entry->d_name);
if (stat(buf, &sb) == -1) {
perror("stat");
continue;
}
printf("%c%c%c%c%c%c%c%c%c%c %ld ",
S_ISDIR(sb.st_mode) ? 'd' : '-',
sb.st_mode & S_IRUSR ? 'r' : '-',
sb.st_mode & S_IWUSR ? 'w' : '-',
sb.st_mode & S_IXUSR ? 'x' : '-',
sb.st_mode & S_IRGRP ? 'r' : '-',
sb.st_mode & S_IWGRP ? 'w' : '-',
sb.st_mode & S_IXGRP ? 'x' : '-',
sb.st_mode & S_IROTH ? 'r' : '-',
sb.st_mode & S_IWOTH ? 'w' : '-',
sb.st_mode & S_IXOTH ? 'x' : '-',
sb.st_nlink);
struct passwd *pw = getpwuid(sb.st_uid);
if (pw != NULL) {
printf("%s ", pw->pw_name);
} else {
printf("%d ", sb.st_uid);
}
struct group *gr = getgrgid(sb.st_gid);
if (gr != NULL) {
printf("%s ", gr->gr_name);
} else {
printf("%d ", sb.st_gid);
}
printf("%ld ", sb.st_size);
struct tm *tm = localtime(&sb.st_mtime);
char time_buf[32];
strftime(time_buf, sizeof(time_buf), "%Y-%m-%d %H:%M:%S", tm);
printf("%s ", time_buf);
printf("%s\n", entry->d_name);
}
closedir(dir);
exit(EXIT_SUCCESS);
}
```
该程序使用 `opendir()` 和 `readdir()` 函数打开并读取指定目录中的所有文件和子目录,然后对每个文件(夹)使用 `stat()` 函数获取其属性信息并打印出来。对于文件类型,程序根据 `st_mode` 字段中的 `S_ISDIR()` 宏来判断是否为目录。对于权限,程序使用 `st_mode` 字段中的各个标志位来判断是否具有读、写和执行权限。对于所有者用户名和所在组用户名,程序使用 `getpwuid()` 和 `getgrgid()` 函数分别获取其对应的 `passwd` 和 `group` 结构体,并从中获取用户名。对于文件大小,程序使用 `st_size` 字段。对于最后修改时间,程序使用 `st_mtime` 字段,并使用 `localtime()` 和 `strftime()` 函数将其格式化为字符串。最后,程序打印出文件(夹)名。
linux用C语言编程,打开etc/passwd文件,找出已经注册的普通用户账号
可以使用以下代码来实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LENGTH 1024
int main() {
FILE *file = fopen("/etc/passwd", "r");
if (file == NULL) {
printf("Failed to open file\n");
return 1;
}
char line[MAX_LINE_LENGTH];
while (fgets(line, MAX_LINE_LENGTH, file)) {
char *username = strtok(line, ":");
char *password = strtok(NULL, ":");
char *uid = strtok(NULL, ":");
char *gid = strtok(NULL, ":");
char *comment = strtok(NULL, ":");
char *home = strtok(NULL, ":");
char *shell = strtok(NULL, ":");
if (atoi(uid) >= 1000 && atoi(uid) < 65534) {
printf("%s\n", username);
}
}
fclose(file);
return 0;
}
```
该代码会打开 `/etc/passwd` 文件,并逐行读取。对于每行,它会使用 `strtok` 函数来将其拆分为多个字段。然后它会检查 UID 是否在正确的范围内(通常只有普通用户的 UID 在 1000 到 65533 之间),并打印出相应的用户名。
阅读全文
相关推荐
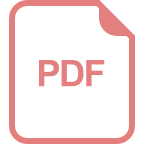
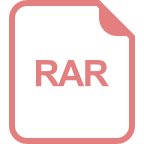
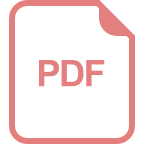
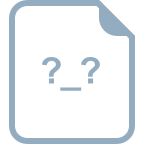
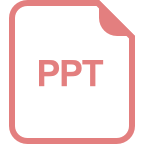
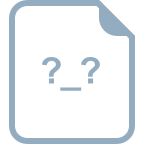
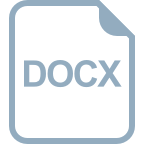
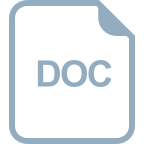
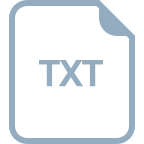
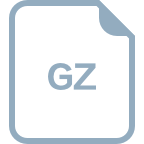
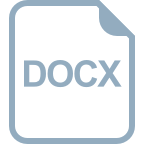
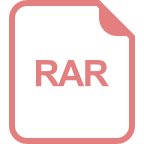
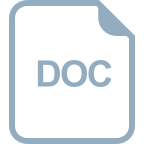
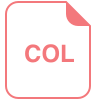
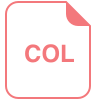
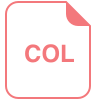
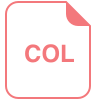