利用python中的tkinter设计gui,结合mysql数据库,编写外卖商家收入金额统计功能,其中商品得到成本与与售价都在数据库中给出
时间: 2024-03-02 22:54:05 浏览: 22
实现这个功能,需要考虑以下几个步骤:
1. 创建MySQL数据库,用于存储商品的成本和售价等信息。可以使用Python中的pymysql模块连接MySQL数据库并进行操作。
2. 使用tkinter设计GUI界面,包括输入框、按钮、标签等控件,用于输入查询条件和显示查询结果。
3. 编写Python代码,实现查询和计算商家收入金额的功能。可以先从MySQL数据库中读取商品的成本和售价等信息,然后根据用户输入的查询条件,筛选出符合条件的商品信息,最后计算商家的收入金额并在GUI界面中显示。
下面是一个简单的Python代码示例,实现了上述功能:
```
import pymysql
import tkinter as tk
# 连接MySQL数据库
conn = pymysql.connect(host='localhost', user='root', password='123456', database='test')
cursor = conn.cursor()
# 查询商家收入金额
def calculate_income():
# 从界面中获取查询条件
start_date = start_date_entry.get()
end_date = end_date_entry.get()
# 从数据库中查询符合条件的商品信息
sql = "SELECT * FROM goods WHERE date BETWEEN '{}' AND '{}'".format(start_date, end_date)
cursor.execute(sql)
results = cursor.fetchall()
# 计算商家收入金额
income = 0
for row in results:
cost = row[1]
price = row[2]
quantity = row[3]
income += price * quantity - cost * quantity
# 在界面中显示商家收入金额
income_label.config(text="商家收入金额:{}".format(income))
# 创建GUI界面
root = tk.Tk()
root.title("外卖商家收入金额统计")
start_date_label = tk.Label(root, text="起始日期:")
start_date_label.grid(row=0, column=0)
start_date_entry = tk.Entry(root)
start_date_entry.grid(row=0, column=1)
end_date_label = tk.Label(root, text="截止日期:")
end_date_label.grid(row=1, column=0)
end_date_entry = tk.Entry(root)
end_date_entry.grid(row=1, column=1)
calculate_button = tk.Button(root, text="计算收入", command=calculate_income)
calculate_button.grid(row=2, column=0)
income_label = tk.Label(root)
income_label.grid(row=2, column=1)
root.mainloop()
# 关闭数据库连接
cursor.close()
conn.close()
```
在这个示例代码中,我们先通过pymysql模块连接MySQL数据库,并在GUI界面中创建了两个输入框,用于输入起始日期和截止日期。当用户点击“计算收入”按钮时,程序会从输入框中获取查询条件,并根据条件从数据库中查询符合条件的商品信息。然后,程序会遍历查询结果,计算商家的收入金额,并在GUI界面中显示。
需要注意的是,这只是一个简单的示例代码,实际中还需要考虑更多的细节问题,如异常处理、数据格式转换、界面布局等。
相关推荐
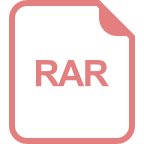
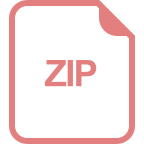








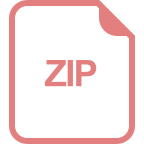
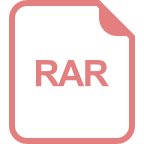
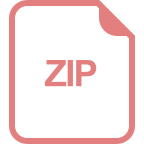
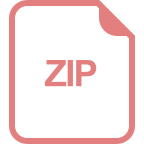
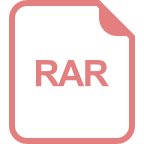
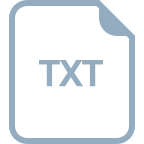
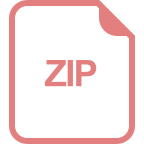