利用python的tkinter进行gui设计,结合数据库mysql写一段代码,实现外卖商家管理模块,这个模块有查询商品详情、新建商品、修改原有商品、删除商品四个功能。其中左侧是商品详情区域,展示所有本店铺的商品信息。右上方是输入区,输入对应功能需要的信息,右下方是功能选择区域,点击其中的按钮会读取上方对应的信息并做对应的操作。对商品鹅新建、修改与删除斗眼写入数据库
时间: 2024-02-23 19:58:21 浏览: 65
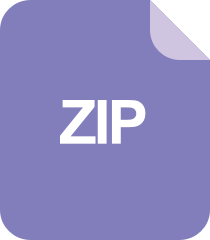
基于python+tkinter+mysql实现外卖数据库管理系统源码.zip
以下是一个简单的示例代码,实现了您所描述的功能:
```python
import tkinter as tk
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
# 创建GUI
root = tk.Tk()
root.title("外卖商家管理模块")
# 查询商品详情功能
def query_details():
# 查询数据库中的商品信息
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM products")
myresult = mycursor.fetchall()
# 清空商品详情区域
detail_area.delete("1.0", tk.END)
# 显示商品信息
for x in myresult:
detail_area.insert(tk.END, "ID: " + str(x[0]) + ", " + x[1] + ", 价格:" + str(x[2]) + ", 库存:" + str(x[3]) + "\n")
# 新建商品功能
def add_product():
# 从输入区域获取商品信息
name = name_entry.get()
price = price_entry.get()
stock = stock_entry.get()
# 插入商品信息到数据库
mycursor = mydb.cursor()
sql = "INSERT INTO products (name, price, stock) VALUES (%s, %s, %s)"
val = (name, price, stock)
mycursor.execute(sql, val)
mydb.commit()
# 清空输入区域
name_entry.delete(0, tk.END)
price_entry.delete(0, tk.END)
stock_entry.delete(0, tk.END)
# 修改商品功能
def modify_product():
# 从输入区域获取商品信息
id = id_entry.get()
name = name_entry.get()
price = price_entry.get()
stock = stock_entry.get()
# 更新数据库中的商品信息
mycursor = mydb.cursor()
sql = "UPDATE products SET name = %s, price = %s, stock = %s WHERE id = %s"
val = (name, price, stock, id)
mycursor.execute(sql, val)
mydb.commit()
# 清空输入区域
id_entry.delete(0, tk.END)
name_entry.delete(0, tk.END)
price_entry.delete(0, tk.END)
stock_entry.delete(0, tk.END)
# 删除商品功能
def delete_product():
# 从输入区域获取商品ID
id = id_entry.get()
# 从数据库中删除商品
mycursor = mydb.cursor()
sql = "DELETE FROM products WHERE id = %s"
val = (id,)
mycursor.execute(sql, val)
mydb.commit()
# 清空输入区域
id_entry.delete(0, tk.END)
# 创建商品详情区域
detail_area = tk.Text(root, width=50, height=20)
detail_area.pack(side=tk.LEFT)
query_details()
# 创建输入区域
input_frame = tk.Frame(root)
input_frame.pack(side=tk.TOP)
id_label = tk.Label(input_frame, text="商品ID:")
id_label.grid(row=0, column=0)
id_entry = tk.Entry(input_frame)
id_entry.grid(row=0, column=1)
name_label = tk.Label(input_frame, text="商品名称:")
name_label.grid(row=1, column=0)
name_entry = tk.Entry(input_frame)
name_entry.grid(row=1, column=1)
price_label = tk.Label(input_frame, text="商品价格:")
price_label.grid(row=2, column=0)
price_entry = tk.Entry(input_frame)
price_entry.grid(row=2, column=1)
stock_label = tk.Label(input_frame, text="商品库存:")
stock_label.grid(row=3, column=0)
stock_entry = tk.Entry(input_frame)
stock_entry.grid(row=3, column=1)
# 创建功能选择区域
button_frame = tk.Frame(root)
button_frame.pack(side=tk.BOTTOM)
query_button = tk.Button(button_frame, text="查询商品详情", command=query_details)
query_button.pack(side=tk.LEFT)
add_button = tk.Button(button_frame, text="新建商品", command=add_product)
add_button.pack(side=tk.LEFT)
modify_button = tk.Button(button_frame, text="修改原有商品", command=modify_product)
modify_button.pack(side=tk.LEFT)
delete_button = tk.Button(button_frame, text="删除商品", command=delete_product)
delete_button.pack(side=tk.LEFT)
# 运行GUI
root.mainloop()
```
请注意,这只是一个示例代码,实际应用中还需要进行更多的错误处理和安全性检查,以确保数据的正确性和安全性。
阅读全文
相关推荐
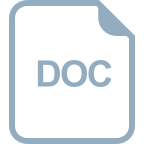
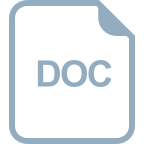



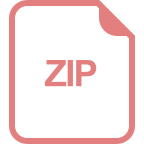
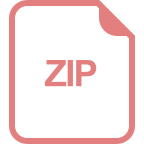





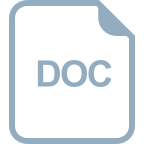
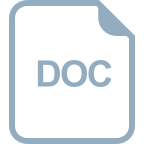
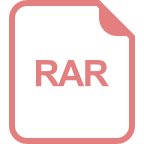