利用python中的tkinter进行gui设计,结合数据库mysql编写一段代码,要求实现顾客操作系统界面,来实现获取正在营业的店铺菜单功能,点外卖提交订单功能,查询历史订单功能。
时间: 2024-02-24 18:56:26 浏览: 22
好的,我可以为您提供一份代码示例,但是需要您提供数据库中的表结构和数据,以便我能够正确地查询和操作数据库。以下是一个简单的示例代码,可以根据您的需求进行修改和扩展:
```python
import tkinter as tk
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
passwd="yourpassword",
database="yourdatabase"
)
# 创建主窗口
root = tk.Tk()
root.title("顾客操作系统")
# 创建标签和文本框
label_shop = tk.Label(root, text="请选择店铺:")
label_shop.pack()
entry_shop = tk.Entry(root)
entry_shop.pack()
label_order = tk.Label(root, text="订单详情:")
label_order.pack()
entry_order = tk.Entry(root)
entry_order.pack()
# 查询菜单函数
def get_menu():
# 获取店铺名称
shop_name = entry_shop.get()
# 查询菜单
cursor = mydb.cursor()
cursor.execute("SELECT * FROM menu WHERE shop=%s", (shop_name,))
menu = cursor.fetchall()
# 显示菜单
for item in menu:
label_item = tk.Label(root, text=item[0] + ": " + str(item[1]))
label_item.pack()
# 提交订单函数
def submit_order():
# 获取订单详情
order_detail = entry_order.get()
# 插入订单
cursor = mydb.cursor()
cursor.execute("INSERT INTO orders (detail) VALUES (%s)", (order_detail,))
mydb.commit()
# 提示订单提交成功
tk.messagebox.showinfo(title="提示", message="订单提交成功!")
# 查询历史订单函数
def get_history():
# 查询历史订单
cursor = mydb.cursor()
cursor.execute("SELECT * FROM orders")
orders = cursor.fetchall()
# 显示历史订单
for item in orders:
label_item = tk.Label(root, text=item[0] + ": " + item[1])
label_item.pack()
# 创建按钮
button_menu = tk.Button(root, text="获取菜单", command=get_menu)
button_menu.pack()
button_order = tk.Button(root, text="提交订单", command=submit_order)
button_order.pack()
button_history = tk.Button(root, text="查询历史订单", command=get_history)
button_history.pack()
# 运行主循环
root.mainloop()
```
需要注意的是,这只是一个简单的示例,您需要根据实际需求对其进行修改和扩展,尤其是对数据库查询和操作的部分需要格外谨慎。
相关推荐
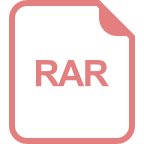
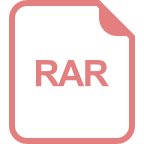














