用python写一个替换文件中指定字符的代码
时间: 2024-05-01 19:16:56 浏览: 12
以下是一个简单的Python代码,用于替换文件中指定字符:
```python
import os
# 定义替换函数
def replace_file_content(file_path, old_str, new_str):
# 打开文件
with open(file_path, 'r') as f:
content = f.read()
# 替换字符
content = content.replace(old_str, new_str)
# 保存文件
with open(file_path, 'w') as f:
f.write(content)
# 替换文件中的指定字符
file_path = 'example.txt'
old_str = 'Hello'
new_str = 'Hi'
replace_file_content(file_path, old_str, new_str)
```
首先,我们定义了一个名为replace_file_content()的函数,该函数接受三个参数:文件路径、要替换的旧字符串和要替换为的新字符串。
在函数中,我们使用Python的内置函数open()打开文件,并使用read()方法读取文件内容。然后,我们使用replace()方法替换旧字符串为新字符串。最后,我们使用write()方法将更新后的文件内容写回原文件中。
最后,我们使用示例文件example.txt和旧字符串'Hello'和新字符串'Hi'来调用这个函数。
相关问题
写一个python代码,批量替换txt文件中指定行的内容
好的,以下是代码实现:
filename = input("请输入文件名:")
with open(filename, 'w') as f:
while True:
string = input("请输入字符串:")
if string == '@':
break
f.write(string)
执行代码后,会提示输入文件名,输入后按回车键确认。然后会提示输入字符串,输入完一个字符串后按回车键确认,直到输入一个@为止,程序会自动结束并将输入的字符串写入指定的文件中。
python文件替换指定字符串
Python可以使用文件IO操作以及字符串处理来实现文件中指定字符串的替换。下面是一个简单的示例。
假设我们有一个文本文件"test.txt",内容如下:
```
Hello World, I am learning Python.
Python is a powerful programming language.
```
我们将使用Python代码来替换其中的字符串"Python"为"Java"。
首先,我们可以打开文件,并读取其内容:
```
file = open("test.txt", "r")
content = file.read()
file.close()
```
接下来,我们使用字符串的replace()方法来替换指定的字符串:
```
new_content = content.replace("Python", "Java")
```
最后,我们将替换后的内容写回文件中:
```
new_file = open("test.txt", "w")
new_file.write(new_content)
new_file.close()
```
完整的代码如下:
```python
file = open("test.txt", "r")
content = file.read()
file.close()
new_content = content.replace("Python", "Java")
new_file = open("test.txt", "w")
new_file.write(new_content)
new_file.close()
```
运行以上代码后,我们的文本文件"test.txt"将会变为:
```
Hello World, I am learning Java.
Java is a powerful programming language.
```
通过以上代码,我们成功替换了文件中的指定字符串。需要注意的是,这仅仅是一个简单的示例,实际应用中可能需要考虑更多的异常处理和边界情况。
相关推荐
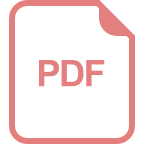
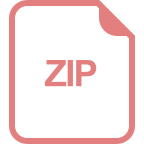
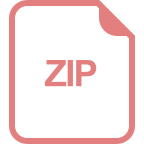












