用线程信号量实现线程同步 1. main 函数中创建 4 个线程,然后等待 4 个线程结束后退出 2. 每个线程执行过程如下: 输出 Pi begin... 等待 1s 输出...Pi done
时间: 2024-05-07 10:21:58 浏览: 6
以下是使用线程信号量实现线程同步的示例代码:
```c++
#include <iostream>
#include <thread>
#include <chrono>
#include <mutex>
#include <condition_variable>
using namespace std;
const int thread_count = 4;
int finished_threads = 0;
mutex mtx;
condition_variable cv;
void thread_func(int id) {
cout << "P" << id << " begin..." << endl;
// 等待 1s
this_thread::sleep_for(chrono::seconds(1));
cout << "...P" << id << " done" << endl;
// 线程结束时通知 main 函数
unique_lock<mutex> lock(mtx);
finished_threads++;
if (finished_threads == thread_count) {
cv.notify_one();
}
}
int main() {
thread threads[thread_count];
// 创建 4 个线程
for (int i = 0; i < thread_count; i++) {
threads[i] = thread(thread_func, i);
}
// 等待所有线程结束
unique_lock<mutex> lock(mtx);
cv.wait(lock, []{ return finished_threads == thread_count; });
// 所有线程结束后退出
for (int i = 0; i < thread_count; i++) {
threads[i].join();
}
return 0;
}
```
在该代码中,我们使用了一个互斥锁和一个条件变量来实现线程同步。在每个线程结束时,我们会将 finished_threads 计数器加 1,并检查是否所有线程都已结束。如果是,则通过条件变量通知主线程。
在主线程中,我们使用 unique_lock 对互斥锁进行加锁,并在条件变量的 wait 函数中等待所有线程结束的通知。一旦收到通知,我们就可以安全地退出程序并等待所有线程结束。
值得注意的是,我们在每个线程结束时对 finished_threads 计数器进行加锁,以避免多个线程同时修改计数器的问题。
相关推荐
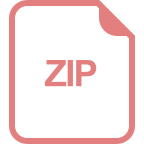
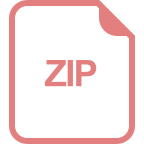
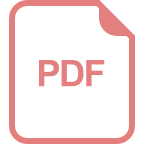














