@RequestMapping(value = "/searchUser", method = RequestMethod.POST) @ResponseBody public ModelAndView searchUser(HttpServletRequest request, User user) { ModelAndView mv = new ModelAndView(); int pageNum = 1; int pageSize = 10; int total = userService.getUserNum(); String phone = user.getPhone(); String username = user.getUsername(); String qq = user.getQq(); List<User> rows = userService.getPageUserByUser(phone, username, qq, pageNum, pageSize); UserGrid userGrid = new UserGrid(); User searchuser = new User(); searchuser.setPhone(phone); searchuser.setUsername(username); searchuser.setQq(qq); userGrid.setCurrent(pageNum); userGrid.setRowCount(pageSize); userGrid.setRows(rows); userGrid.setTotal(total); mv.addObject("userGrid", userGrid); mv.addObject("searchuser", searchuser); mv.setViewName("admin/user/user_list"); return mv; }
时间: 2024-02-02 18:03:01 浏览: 69
这是一段 Java 代码,用于处理一个 POST 请求,请求的路径是 "/searchUser"。代码中使用了 Spring MVC 框架提供的注解 @RequestMapping 和 @ResponseBody,表明这个方法是一个控制器方法,并且返回的是一个 JSON 格式的数据。在方法中,通过 HttpServletRequest 获取请求中的参数,然后调用 userService 中的方法来查询符合条件的用户,并将查询结果封装成一个 UserGrid 对象返回。最后将 UserGrid 对象和查询条件对象 searchuser 添加到 ModelAndView 中,设置视图名称为 "admin/user/user_list" 并返回。
相关问题
@Controller @RequestMapping(value = "/JzAtlas") @Api(tags = {"建筑--图册--操作接口"}) public class JzAtlasAction { @Resource private JzAtlasService service; @ApiOperation(value = "查询单个项目", notes = "项目主键ID") //@RequestMapping(value = "/findByKey", method = {RequestMethod.GET}, produces = "application/json; charset=UTF-8") @RequestMapping(value = "/findByKey", method = {RequestMethod.GET}) @ResponseBody public Object findByKey(Integer id) { return service.findById(id); } @ApiOperation(value = "保存单个项目信息", notes = "传递数据") @RequestMapping(value = "/save", method = {RequestMethod.POST}) @ResponseBody public Object save(@RequestBody JzAtlas model) { return service.save(model); } @ApiOperation(value = "更新单个项目信息", notes = "传递数据") @RequestMapping(value = "/update", method = {RequestMethod.POST}) @ResponseBody public Object update(@RequestBody JzAtlas model) { return service.update(model); } @ApiOperation(value = "查询所有", notes = "无查询条件") @RequestMapping(value = "/findAllList", method = {RequestMethod.POST}) @ResponseBody public Object findList() { return service.findList(); } @ApiOperation(value = "根据条件查询", notes = "查询参数") @RequestMapping(value = "/findList", method = {RequestMethod.POST}) @ResponseBody public Object findListByQuery(@RequestBody JzAtlasRequest requestModel) { return service.findListByQuery(requestModel); } @ApiOperation(value = "删除单个项目信息", notes = "项目主键ID") @RequestMapping(value = "/delete", method = {RequestMethod.POST}) @ResponseBody public Object delete(Integer id) { return service.delete(id); } }
这是一个Java类,使用了Spring框架的@Controller注解,表示这是一个控制器类。通过@RequestMapping注解指定了处理请求的URL路径为"/JzAtlas"。@Api注解用于生成API文档,其中的tags属性指定了文档中的标签为"建筑--图册--操作接口"。
该类中包含了多个处理请求的方法,使用了不同的请求方法(GET、POST)和URL路径。每个方法都使用了@ResponseBody注解,表示返回的结果将被转换为JSON格式。其中,@ApiOperation注解用于生成API文档中的方法描述。
- findByKey方法:用于根据项目主键ID查询单个项目信息。
- save方法:用于保存单个项目信息,接收一个JzAtlas对象作为参数。
- update方法:用于更新单个项目信息,接收一个JzAtlas对象作为参数。
- findList方法:用于查询所有项目信息。
- findListByQuery方法:根据条件查询项目信息,接收一个JzAtlasRequest对象作为参数。
- delete方法:删除单个项目信息,接收一个项目主键ID作为参数。
其中,@Resource注解用于注入JzAtlasService对象,表示该类依赖于JzAtlasService服务类。
使用ResponseEntity<>改写以下代码:@Controller @RequestMapping("/user") public class UserController { @RequestMapping(value = "/login", method = RequestMethod.POST) public ModelAndView login(User user,HttpSession httpSession) { ModelAndView modelAndView = new ModelAndView(); if (user.getUserName().equals("张三") && user.getPassword().equals("123")) { //modelAndView.addObject("user", user); httpSession.setAttribute("user",user); modelAndView.setViewName("success"); } else { modelAndView.setViewName("fail"); } return modelAndView; } }
@Controller
@RequestMapping("/user")
public class UserController {
@PostMapping("/login")
public ResponseEntity<String> login(User user, HttpSession httpSession) {
if (user.getUserName().equals("张三") && user.getPassword().equals("123")) {
httpSession.setAttribute("user", user);
return ResponseEntity.ok("success");
} else {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).body("fail");
}
}
}
注意,这里返回的是一个ResponseEntity<String>对象,其中泛型指定了返回值的类型为String。如果登录成功,使用ResponseEntity.ok()返回一个状态码为200的响应,并将成功信息放入响应体;如果登录失败,使用ResponseEntity.status(HttpStatus.UNAUTHORIZED)返回一个状态码为401的响应,并将失败信息放入响应体。
阅读全文
相关推荐
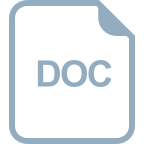
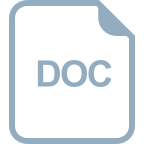
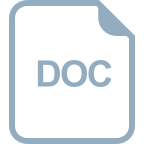














