用python编写循迹小车
时间: 2023-07-17 07:59:31 浏览: 169
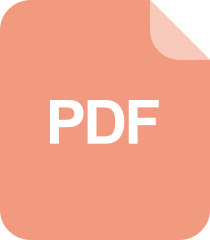
循迹小车编程方法
当使用Python编写循迹小车时,你可以使用树莓派或其他支持Python的单板计算机来控制小车的GPIO引脚。以下是一个简单的示例代码,演示了如何使用GPIO库控制循迹小车:
```python
import RPi.GPIO as GPIO
import time
# 定义引脚号
left_motor_pin1 = 17
left_motor_pin2 = 18
right_motor_pin1 = 27
right_motor_pin2 = 22
# 初始化GPIO
GPIO.setmode(GPIO.BCM)
GPIO.setup(left_motor_pin1, GPIO.OUT)
GPIO.setup(left_motor_pin2, GPIO.OUT)
GPIO.setup(right_motor_pin1, GPIO.OUT)
GPIO.setup(right_motor_pin2, GPIO.OUT)
# 前进
def forward():
GPIO.output(left_motor_pin1, GPIO.HIGH)
GPIO.output(left_motor_pin2, GPIO.LOW)
GPIO.output(right_motor_pin1, GPIO.HIGH)
GPIO.output(right_motor_pin2, GPIO.LOW)
# 后退
def backward():
GPIO.output(left_motor_pin1, GPIO.LOW)
GPIO.output(left_motor_pin2, GPIO.HIGH)
GPIO.output(right_motor_pin1, GPIO.LOW)
GPIO.output(right_motor_pin2, GPIO.HIGH)
# 左转
def left():
GPIO.output(left_motor_pin1, GPIO.LOW)
GPIO.output(left_motor_pin2, GPIO.HIGH)
GPIO.output(right_motor_pin1, GPIO.HIGH)
GPIO.output(right_motor_pin2, GPIO.LOW)
# 右转
def right():
GPIO.output(left_motor_pin1, GPIO.HIGH)
GPIO.output(left_motor_pin2, GPIO.LOW)
GPIO.output(right_motor_pin1, GPIO.LOW)
GPIO.output(right_motor_pin2, GPIO.HIGH)
# 停止
def stop():
GPIO.output(left_motor_pin1, GPIO.LOW)
GPIO.output(left_motor_pin2, GPIO.LOW)
GPIO.output(right_motor_pin1, GPIO.LOW)
GPIO.output(right_motor_pin2, GPIO.LOW)
# 主程序
if __name__ == '__main__':
try:
while True:
forward() # 前进
time.sleep(2)
stop() # 停止
time.sleep(1)
backward() # 后退
time.sleep(2)
stop() # 停止
time.sleep(1)
left() # 左转
time.sleep(1)
stop() # 停止
time.sleep(1)
right() # 右转
time.sleep(1)
stop() # 停止
time.sleep(1)
except KeyboardInterrupt:
GPIO.cleanup()
```
上述代码使用RPi.GPIO库来控制GPIO引脚,通过定义不同的函数来控制小车的运动方向(前进、后退、左转、右转、停止)。在主程序中,使用无限循环来不断执行不同的运动操作。当按下键盘上的Ctrl+C时,会捕获KeyboardInterrupt异常,然后执行GPIO.cleanup()来清理GPIO引脚的设置。请注意,引脚号和具体的引脚连接方式可能会有所不同,需要根据实际情况进行调整。
阅读全文
相关推荐

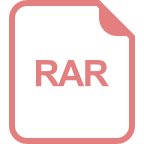
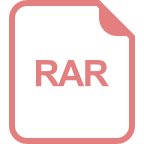
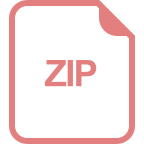
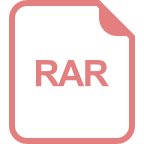
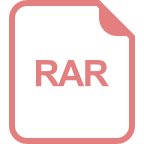
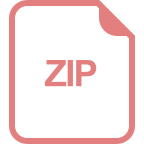
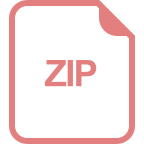
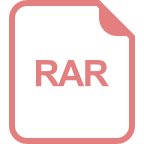
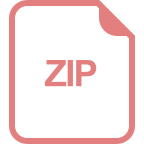
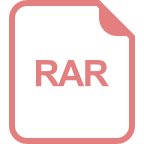



