class B{ int x = 100, y = 200; public void setX (int x){ x = x; } public void setY (int y) { this. y = y; } public int getXYSum () { return x+y; } } public class E { public static void main (String args []) { B b = new B(); b. setX (-100) ; b. setY (88); System. out. printf ("%d:%d:%d", b. x, b. y, b. getXYSum ()) ; } }详细解读该段代码
时间: 2024-02-10 14:32:42 浏览: 72
这段代码定义了一个类 B,包含了两个属性 x 和 y,以及三个方法 setX、setY 和 getXYSum。
在类 B 中,setX 方法接受一个 int 类型的参数 x,但是方法内部的 x 变量和类的属性 x 重名了,这就导致了方法内部的 x 变量会遮盖类的属性 x,所以在方法内部对 x 进行修改并不会影响类的属性 x,因此,在 setX 方法内部,x = x; 并没有起到修改 x 属性值的作用。正确的做法应该是使用 this 关键字来引用类的属性 x,即 this.x = x;。
setY 方法接受一个 int 类型的参数 y,使用 this 关键字来引用类的属性 y,并将传入的参数值赋值给 y 属性。
getXYSum 方法没有参数,返回 x 和 y 属性值的和。
在类 B 的外部,E 类的 main 方法中首先创建了一个 B 类的对象 b,然后分别调用 b 的 setX 和 setY 方法,将 x 和 y 属性的值修改为 -100 和 88。最后打印出 b 的 x、y 和 getXYSum 方法的返回值,分别为 -100、88 和 -12(因为 x 和 y 的值已经被修改为 -100 和 88,所以它们的和为 -12)。
相关问题
class B[int x=100,y=200:public void setX(int x)[X =X; public void setY(int y)[this.y=y; public int getXYSum()[return xty public class A[public static void main(String args[])[Bb=newBOb.setX(-100);b.setY(-200)System.out.printf("sum="+b.getXYSum))
根据您提供的代码,类B具有以下方法和属性:
- 方法setX(int x):将x的值赋给B类的属性x。
- 方法setY(int y):将y的值赋给B类的属性y。
- 方法getXYSum():返回属性x和属性y的和。
- 属性x:类型为整数,初始值为100。
- 属性y:类型为整数,初始值为200。
类A中的main方法创建了一个B类的实例b,并通过调用setX(-100)和setY(-200)方法将x和y的值设置为-100和-200。然后,通过调用b.getXYSum()方法获取x和y的和,并使用System.out.printf()方法打印输出结果。
请注意,您提供的代码中存在一些语法错误,例如缺少分号、括号不匹配等。修正这些错误后,代码应该如下所示:
```java
class B {
int x = 100;
int y = 200;
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
public int getXYSum() {
return x + y;
}
}
public class A {
public static void main(String[] args) {
B b = new B();
b.setX(-100);
b.setY(-200);
System.out.printf("sum = %d", b.getXYSum());
}
}
```
这样,运行代码将会输出"sum = -300"作为结果。
class B{ int x=100,y=200; public void setx(int x){ x=x; } public void sety(int y){ this.y=y; } public int getxYsum(){ return x+y; } } public class A{ public static void main (String args[]){ B b=new B(); b.setx(-100); b.sety(-200); System.out.printin( "sum="+b.getXYSum()); } }
这段代码存在一个错误,即在类B中的setx方法中,使用了与该方法参数同名的变量x,导致在方法内部无法正确修改类成员变量x的值。正确的setx方法应该像这样:
```
public void setx(int newX){
x = newX;
}
```
这样,在调用`b.setx(-100)`时,类成员变量x的值会被正确修改为-100,最终输出的sum应该为-300。
阅读全文
相关推荐
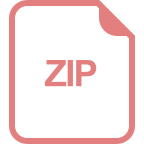
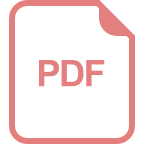
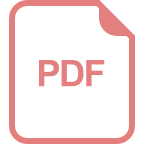












