For the following C statement,write the corresponding RISC-V assembly code.Assume that the C variables f,g,and h,have already been placed in registers x5,x6,and x7 respectively.Use a minimal number of RISC-V assemblyinstructions.
时间: 2024-02-21 19:01:54 浏览: 26
C statement:
```c
f = (g + h) << 2;
```
RISC-V assembly code:
```assembly
addw x5, x6, x7 # 将 g 和 h 相加并将结果放入 x5 寄存器
slli x5, x5, 2 # 将 x5 寄存器的值左移 2 位,相当于乘以 4
```
Explanation:
首先,我们将 g 和 h 相加并将结果存储在 x5 中,使用 addw 指令。
然后,我们将 x5 寄存器的值左移 2 位,使用 slli 指令。这相当于将结果乘以 4,因为左移 2 位相当于乘以 2 的平方。最终结果存储在 f 变量中,即 x5 寄存器中。
相关问题
For the following C statement, write the corresponding RISC-V assembly code. Assume that the variables f, g, h, i, and j are assigned to registers x5, x6, x7, x28, and x29, respectively. Assume that the base address of the arrays A and B are in registers x10 and x11, respectively. B[8] = A[i−j];
C statement:
```c
B[8] = A[i - j];
```
RISC-V assembly code:
```assembly
subw x8, x28, x29 # 计算 i-j 并将结果放入 x8 寄存器
slli x8, x8, 2 # 将 x8 寄存器的值左移 2 位,即乘以 4
add x8, x8, x10 # 将 x8 寄存器的值(即 A[i-j] 的地址)与 x10 寄存器中的偏移量相加,得到 A[i-j] 的地址
lw x9, 32(x8) # 从 A[i-j] 的地址开始,读取 4 字节(即一个 int 类型的值),并将其存储在 x9 寄存器中
sw x9, 32(x11) # 将 x9 寄存器中的值存储到 B[8] 的地址处
```
Explanation:
首先,我们计算 i-j 的值并将结果存储在 x8 寄存器中,使用 subw 指令。
然后,我们将 x8 寄存器的值左移 2 位,相当于乘以 4,使用 slli 指令。这是因为每个 int 类型的变量占用 4 个字节,因此我们需要将偏移量乘以 4 以获取正确的地址偏移量。
接下来,我们将 x8 寄存器的值(即 A[i-j] 的地址)与数组 A 的基地址相加,使用 add 指令,以获取数组元素 A[i-j] 的地址。
然后,我们使用 lw 指令从 A[i-j] 的地址开始读取 4 字节(即一个 int 类型的值),并将其存储在 x9 寄存器中。
最后,我们使用 sw 指令将 x9 寄存器中的值存储到数组 B 中下标为 8 的元素处,即 B[8]。注意,我们将数组 B 的基地址存储在了寄存器 x11 中。
For the RISC-V assembly instructions below, what is the corresponding C statement? Assume that the variables f, g, h, i, and j are assigned to registers x5, x6, x7, x28, and x29, respectively. Assume that the base address of the arrays A and B are in registers x10 and x11, respectively. slli x30, x5, 2 // x30 = f*4 add x30, x10, x30 // x30 = &A[f] slli x31, x6, 2 // x31 = g*4 add x31, x11, x31 // x31 = &B[g] lw x5, 0(x30) // f = A[f] addi x12, x30, 4 lw x30, 0(x12) add x30, x30, x5 sw x30, 0(x31)
The corresponding C statement is:
```
B[g+1] = A[f] + A[f+1];
```
Explanation:
- `slli x30, x5, 2`: Shift the value of register `x5` (which contains `f`) left by 2 bits (multiply by 4) and store the result in register `x30`.
- `add x30, x10, x30`: Add the value of register `x10` (which contains the base address of array `A`) to the value of register `x30` (which now contains `&A[f]`) and store the result in register `x30`. Now `x30` contains the memory address of `A[f]`.
- `slli x31, x6, 2`: Shift the value of register `x6` (which contains `g`) left by 2 bits (multiply by 4) and store the result in register `x31`.
- `add x31, x11, x31`: Add the value of register `x11` (which contains the base address of array `B`) to the value of register `x31` (which now contains `&B[g]`) and store the result in register `x31`. Now `x31` contains the memory address of `B[g]`.
- `lw x5, 0(x30)`: Load the value stored at memory address `x30` into register `x5`. Now `x5` contains the value of `A[f]`.
- `addi x12, x30, 4`: Add 4 to the value of register `x30` (which still contains the memory address of `A[f]`) and store the result in register `x12`. Now `x12` contains the memory address of `A[f+1]`.
- `lw x30, 0(x12)`: Load the value stored at memory address `x12` (which now contains `A[f+1]`) into register `x30`.
- `add x30, x30, x5`: Add the value of register `x5` (which contains `A[f]`) to the value of register `x30` (which now contains `A[f+1]`) and store the result in register `x30`. Now `x30` contains the sum `A[f] + A[f+1]`.
- `sw x30, 0(x31)`: Store the value of register `x30` (which contains `A[f] + A[f+1]`) at the memory address `x31` (which contains `B[g+1]`). Now `B[g+1]` is updated with the sum `A[f] + A[f+1]`.
相关推荐
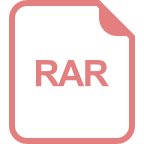
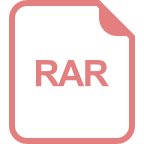
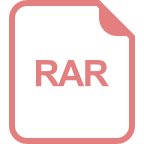












