1、编写Diffie-Hellman程序,协商出用于收、发双方的加、解密密钥K; 2、模拟发送端A,用协商出的密钥K,通过对称加密算法(具体算法不限)将文本文件加密;模拟接收端B,用协商出的密钥K,对接收到的密文解密。
时间: 2024-05-08 13:21:56 浏览: 16
以下是Python实现的Diffie-Hellman密钥交换和对称加密解密的示例代码:
```python
from random import randint
# 定义素数p和原根g
p = 113
g = 3
# 生成随机的私钥a和b
a = randint(1, p-1)
b = randint(1, p-1)
# 计算A和B的公钥
A = pow(g, a, p)
B = pow(g, b, p)
# A和B交换公钥,并计算出共同的密钥K
K = pow(B, a, p)
# K也可以用pow(A, b, p)计算得到,结果相同
# 加密和解密函数
def encrypt(msg, key):
return ''.join([chr((ord(c) + key) % 256) for c in msg])
def decrypt(cipher, key):
return ''.join([chr((ord(c) - key) % 256) for c in cipher])
# 待加密的文本文件
filename = 'plaintext.txt'
# 读取文件内容
with open(filename, 'r') as f:
plaintext = f.read()
# 加密文件内容
cipher = encrypt(plaintext, K)
# 把加密后的密文写入文件
with open('ciphertext.txt', 'w') as f:
f.write(cipher)
# 读取密文文件内容
with open('ciphertext.txt', 'r') as f:
cipher = f.read()
# 解密密文文件内容
plaintext = decrypt(cipher, K)
# 把解密后的明文写入文件
with open('decrypted.txt', 'w') as f:
f.write(plaintext)
```
需要注意的是,加密和解密的算法可以自己根据需求选择,示例代码中使用的是简单的加密方式,不具有实际安全性。
相关推荐
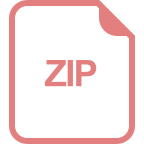















