实现论坛管理,1、在mysql中创建论坛表,包含留言时间、留言IP地址、留言内容,2、在Servlet程序中实现发表留言、留言显示、删除留言
时间: 2024-05-27 10:14:38 浏览: 8
1、在MySQL中创建论坛表:
CREATE TABLE forum (
id INT PRIMARY KEY AUTO_INCREMENT,
time DATETIME,
ip VARCHAR(20),
content VARCHAR(500)
);
2、在Servlet程序中实现发表留言、留言显示、删除留言:
发表留言:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String content = request.getParameter("content");
String ip = request.getRemoteAddr();
Date date = new Date();
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String time = dateFormat.format(date);
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
String sql = "INSERT INTO forum (time, ip, content) VALUES (?, ?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, time);
pstmt.setString(2, ip);
pstmt.setString(3, content);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
response.sendRedirect("forum.jsp");
}
留言显示:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
String sql = "SELECT * FROM forum ORDER BY time DESC";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
List<Forum> list = new ArrayList<>();
while (rs.next()) {
Forum forum = new Forum();
forum.setId(rs.getInt("id"));
forum.setTime(rs.getString("time"));
forum.setIp(rs.getString("ip"));
forum.setContent(rs.getString("content"));
list.add(forum);
}
request.setAttribute("list", list);
request.getRequestDispatcher("forum.jsp").forward(request, response);
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
删除留言:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
String sql = "DELETE FROM forum WHERE id=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, id);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
response.sendRedirect("forum.jsp");
}
相关推荐
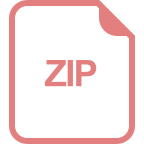
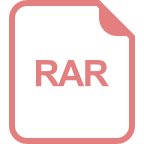
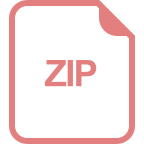








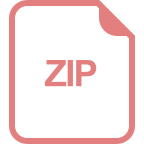
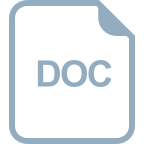
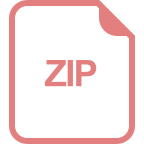
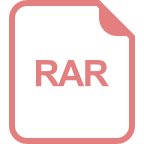
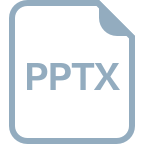
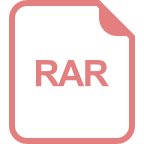
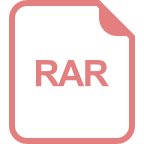