用vue2写一个购物车‘
时间: 2023-05-23 14:05:18 浏览: 112
A:
首先,我们要确定购物车需要哪些功能,比如:
1. 展示商品列表
2. 加入购物车
3. 展示购物车中的商品列表
4. 修改购物车中的商品数量或删除商品
5. 计算商品价格和总价格
接下来,我们可以开始编写代码了。
首先,在 `index.html` 中引入 `vue.js` 和 `app.js`:
```
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Shopping Cart</title>
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
</head>
<body>
<div id="app">
<h1>Shopping Cart</h1>
<div v-if="!showCart">
<ul>
<li v-for="(item, index) in items" :key="index">
<span>{{ item.name }}</span>
<span>${{ item.price }}</span>
<button @click="addToCart(index)">Add to Cart</button>
</li>
</ul>
</div>
<div v-else>
<ul>
<li v-for="(item, index) in cartItems" :key="index">
<span>{{ item.name }}</span>
<span>${{ item.price }}</span>
<button @click="changeQuantity(index, -1)">-</button>
<span>{{ item.quantity }}</span>
<button @click="changeQuantity(index, 1)">+</button>
<button @click="removeFromCart(index)">Remove</button>
</li>
</ul>
<p>Total price: ${{ totalPrice }}</p>
</div>
<button @click="toggleCart">{{ buttonText }}</button>
</div>
<script src="app.js"></script>
</body>
</html>
```
在 `app.js` 中,我们可以定义 Vue 实例并添加组件的属性和方法:
```
const app = new Vue({
el: '#app',
data: {
showCart: false,
buttonText: 'Show Cart',
items: [
{ name: 'item1', price: 10 },
{ name: 'item2', price: 20 },
{ name: 'item3', price: 30 }
],
cartItems: []
},
computed: {
totalPrice() {
return this.cartItems.reduce((acc, cur) => acc + cur.price * cur.quantity, 0);
}
},
methods: {
addToCart(index) {
const item = this.items[index];
const cartItem = this.cartItems.find(itemInCart => itemInCart.name === item.name);
if (cartItem) {
cartItem.quantity++;
} else {
this.cartItems.push({ ...item, quantity: 1 });
}
},
changeQuantity(index, amount) {
const cartItem = this.cartItems[index];
cartItem.quantity += amount;
if (cartItem.quantity === 0) {
this.removeFromCart(index);
}
},
removeFromCart(index) {
this.cartItems.splice(index, 1);
},
toggleCart() {
this.showCart = !this.showCart;
this.buttonText = this.showCart ? 'Hide Cart' : 'Show Cart';
}
}
});
```
在 `data` 中,我们定义了初始状态下的商品列表和购物车列表。`showCart` 表示是否显示购物车界面,`buttonText` 改变按钮的显示文本。我们使用了一个 `computed` 属性 `totalPrice` 来计算购物车中所有商品的总价格。
在 `methods` 中,`addToCart` 将商品添加到购物车中。如果购物车已有该商品,则对应的数量加一;否则将该商品加入购物车,并将数量初始为一。`changeQuantity` 用于增加或减少购物车中某件商品的数量,并在数量减少到零时自动从购物车中删除。`removeFromCart` 用于删除购物车中的商品。`toggleCart` 用于切换是否显示购物车界面。
最后,在 `index.html` 的 CSS 中添加样式:
```
li {
display: flex;
justify-content: space-between;
align-items: center;
}
li button {
margin-left: 10px;
}
#app {
max-width: 600px;
margin: auto;
padding: 20px;
}
```
完成后,即可打开 `index.html` 文件查看购物车页面。
阅读全文
相关推荐
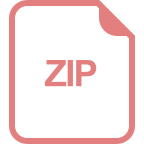












